In today’s digital landscape, web application security is non-negotiable. With cyber threats becoming more sophisticated, relying solely on manual security testing is no longer viable. OWASP ZAP (Zed Attack Proxy) is a powerful, and also an open-source tool that automates security testing, helping you identify vulnerabilities before attackers do. In this blog, we’ll explore how to automate security testing using OWASP ZAP, with a focus on authenticated web application scans.
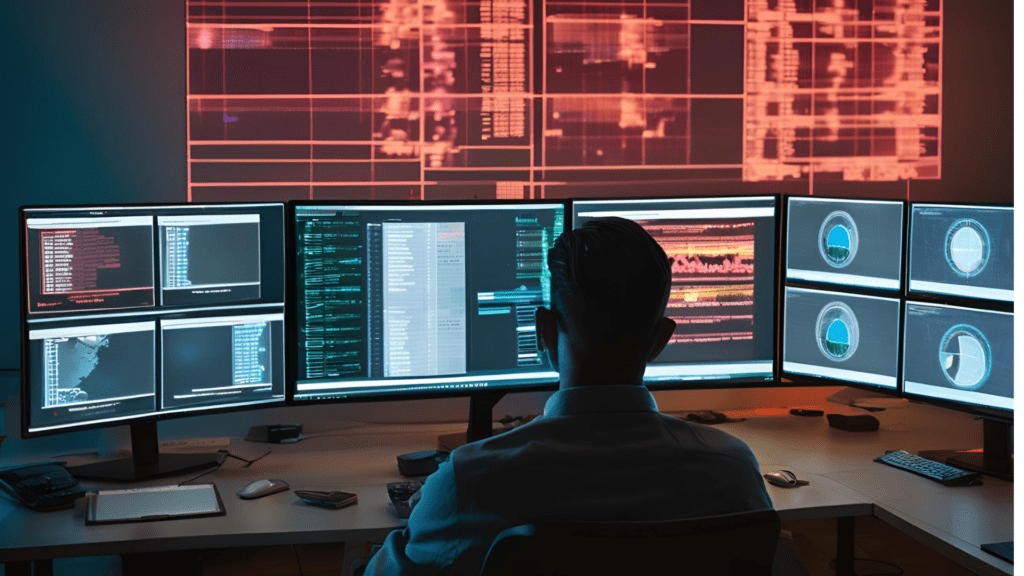
Why to Automate Security Testing?
Manual security testing is time-consuming and prone to human error. Automation offers several advantages:
- Efficiency: Run scans overnight or integrate them into your CI/CD pipeline.
- Accuracy: Automated tools like ZAP can thoroughly scan every part of your application.
- Consistency: Automated tests ensure that the same tests are run every time, reducing the risk of oversight.
- Scalability: Easily scale your security testing efforts across multiple applications or environments.
Getting Started with OWASP ZAP Automation Security Testing Automation with ZAP and Selenium
1. Automating Active Scans with ZAP API
OWASP ZAP provides a robust API that allows you to automate security testing programmatically. Here’s how you can get started:
- ZAP API Basics: The ZAP API is available in JSON, HTML, and XML formats. You can interact with it directly or use client libraries in Python, Java, Shell, and more.
- API URL Format:
http://zap/<formats>/<components>/<operations>/<operation_names[//?<parameters>]
. - Use Cases: You can use the API to explore, attack, scan, authenticate, and generate reports for web applications.
Pro Tip: To prevent API key verification issues, use the -config api.disablekey=true
flag while running ZAP.
2. Automating Scans with YAML Configuration Files
ZAP’s Automation Framework allows you to define your security tests in a YAML configuration file. This approach is flexible and easy to maintain. Here’s what you need to know:
- Basic YAML Template: Define environment variables, contexts, and jobs (e.g., active scan, spider, report generation).
- Example YAML Configuration:
env:
contexts:
- name: "Example Context"
urls:
- <https://example.com>
authentication:
method: 'form'
parameters:
loginPageUrl: "<https://example.com>"
loginRequestUrl: "<login_api>"
loginRequestBody: "<login_request_body>"
jobs:
- type: activeScan
parameters:
context: "Example Context"
Jobs: You can automate tasks like spidering, active scanning, and generating reports. Each job has specific parameters that you can customize.
Reference: https://www.zaproxy.org/docs/automate/automation-framework/
Why Advanced Authenticated Scans Are Crucial?:
Modern web applications often require authentication to access certain resources. Without proper authentication, your security scans may miss critical vulnerabilities. Here’s why advanced authenticated scans are essential:
1. Access to Protected Resources
- Many web applications have protected areas (e.g., user dashboards, admin panels) that are only accessible after authentication.
- Without authenticated scans, these areas remain untested, leaving potential vulnerabilities undetected.
2. Simulating Real User Behavior
- Authenticated scans simulate real user behavior, allowing you to test the application as an actual user would.
- This helps identify vulnerabilities that only appear after logging in, such as session hijacking, CSRF (Cross-Site Request Forgery), and privilege escalation.
3. Comprehensive Coverage
- Authenticated scans ensure that all resources (e.g., APIs, hidden endpoints) are tested, not just the publicly accessible ones.
- This is especially important for applications with role-based access control (RBAC), where different users have access to different parts of the application.
4. Identifying Authentication Flaws
Authenticated scans can help identify flaws in the authentication mechanism itself, such as weak password policies, lack of multi-factor authentication (MFA), or insecure session management
Implementing Authenticated Scans with ZAP:
1. Form-Based Authentication
Most web applications use form-based authentication for login flows. ZAP allows you to automate this security test process using a YAML configuration file. Here’s what you need to include:
- loginPageUrl: The URL of the login page.
- loginRequestUrl: The API endpoint hit during login.
- loginRequestBody: The body of the login request (e.g., username and password).
Example:
authentication:
method: 'form'
parameters:
loginPageUrl: "<https://example.com>"
loginRequestUrl: "<login_api>"
loginRequestBody: "remember_me=true&email=user@example.com&password=Password123"
2. Script-Based Authentication
For more complex authentication mechanisms, you can use script-based authentication. ZAP supports scripting engines like Mozilla Zest, Gradle JS, and Oracle JS. These scripts can be recorded and replayed in ZAP, making it easy to automate even the most intricate login flows.
3. Integrating Zap with the automation framework:
By Integrating the automation framework which is already ensures the actual functional features will include all the resources of a application with the zap.
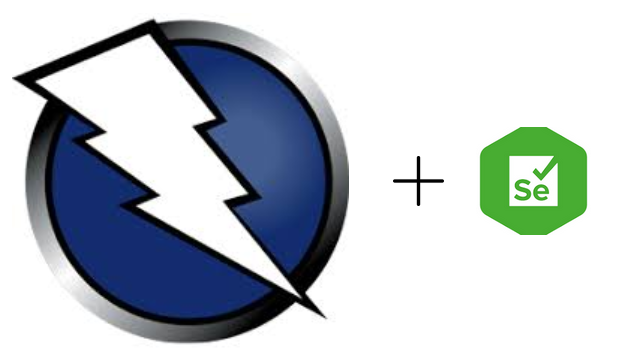
Integrating ZAP with Your Automation Framework
To fully leverage ZAP’s capabilities to perform security test, you can integrate it with your existing automation framework. Here’s how it’s done:
- Proxy Configuration: Redirect all browser traffic to ZAP by configuring the browser driver to use ZAP as a proxy.
Proxy proxy = new Proxy(); proxy.setHttpProxy("localhost:8082"); proxy.setSSLProxy("localhost:8082"); FirefoxOptions options = new FirefoxOptions();
options.setProxy(proxy);
Automation Framework Integration: Use ZAP’s API to start scans, monitor progress, and generate reports. For example, you can create a Seperate
class with methods like startActiveScan
and checkActionCompletion
using the inbuilt methods availabe in the Java Zapapi package.
Selenium Integration:
Prerequsites:
- Selenium
- Zap yaml file configurations for the scope of the security scan.
package ConfigExecution.utility;
import static ConfigExecution.executionEngine.DriverScript.teamName;
import TestCaseExecution.ActionKeyword.*;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.zaproxy.clientapi.core.ApiResponse;
import org.zaproxy.clientapi.core.ClientApi;
public class SecurityTest {
ClientApi api = new ClientApi("localhost", 8080);
public void startActiveScan() {
try{
String YamlFile = teamName+".yaml";
ApiResponse apiResponse =
api.automation.runPlan(
"/home/circleci/project/src/main/resources/DataEngine/" + YamlFile);
String reportPath = checkActionCompletion(Integer.parseInt(String.valueOf(apiResponse)));
if (reportPath != null) {
System.out.println("Report generated at: " + reportPath);
} else {
System.out.println("Failed to generate report.");
}
} catch (Exception e) {
e.printStackTrace();
}
}
public String getReportPath(String jsonResponse) throws JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
JsonNode rootNode = mapper.readTree(jsonResponse);
JsonNode finishedNode = rootNode.path("finished");
if (!finishedNode.isMissingNode()) {
System.out.println("Job is completed.");
JsonNode infoNode = rootNode.path("info");
for (JsonNode item : infoNode.get("items")) {
String value = item.get("value").asText();
if (value.startsWith("Job report generated report")) {
return value.substring(value.indexOf("/"));
}
}
} else {
System.out.println("Job is not completed yet.");
}
return null; // Return null if an error occurs or job is not completed
}
public String checkActionCompletion(int planId) throws Exception {
while (true) {
// Make API request to check progress
URL obj =
new URL("http://localhost:8080/JSON/automation/view/planProgress/?planId=" + planId);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Check if the report is generated
String jsonResponse = response.toString();
Logger logger = LoggerFactory.getLogger("Active scan status");
logger.info(jsonResponse);
if (jsonResponse.contains("Job report finished")) {
return getReportPath(jsonResponse);
} else {
// If report is not finished, wait for some time before checking again
Thread.sleep(3000); // Wait for 5 seconds before checking again
}
} else {
System.out.println("Failed to fetch progress. Response code: " + responseCode);
return null;
}
}
}
}
Challenges and Solutions in ZAP Automation:
While automating security testing with ZAP is powerful, it’s not without its challenges. Here are some common issues and how to address them:
- Authentication Issues: Ensure your YAML configuration correctly defines the login flow and user credentials.
- Resource Unavailability: Make sure ZAP is running and accessible during the scan.
- YAML Configuration Errors: Double-check your YAML file for syntax errors and missing parameters.
- API Key Verification: Disable API key verification using the
config api.disablekey=true
flag.
Sample ZAP Report Generation: What to Expect
After running your automated scans, ZAP generates a detailed report that includes:
- Risk Levels: Info, Low, Medium, High.
- Confidence Levels: False Positive, Low, Medium, High, Confirmed.
- Sections: Site risk counts, response body, alerts, and more.
Example Report Generation Config:
ZAP Scanning Report
- Risk: High
- Confidence: Confirmed
- Alerts: Cross-Site Scripting (Reflected), SQL Injection
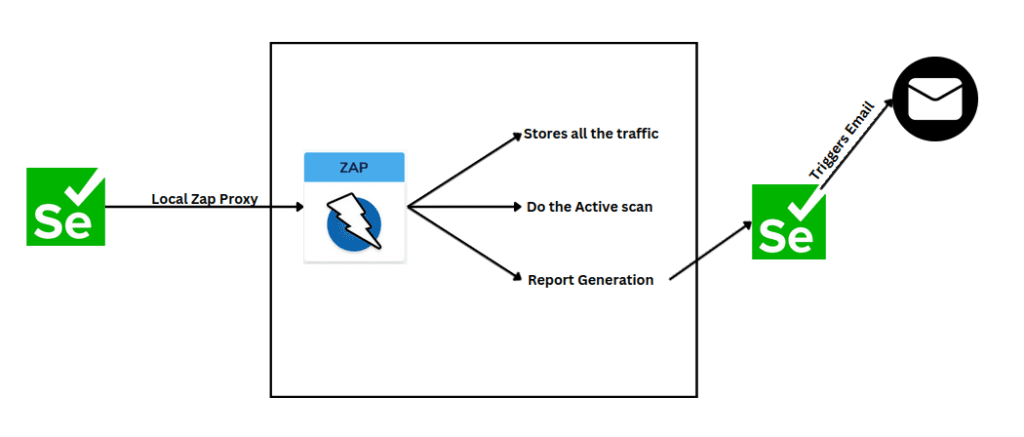
Conclusion: Secure Your Web Applications with ZAP Automation
Automating security testing with OWASP ZAP is a game-changer for developers and security teams. By leveraging ZAP’s API and automation framework, you can ensure comprehensive coverage of your web applications, even for complex authenticated flows. Whether you’re scanning implementing authenticated scans, ZAP provides the tools you need to stay ahead of cyber threats.
So, what are you waiting for? Start automating your security tests with OWASP ZAP today and take your web application security to the next level!
Got questions or need help with ZAP automation? Drop a comment below or reach out to us!
To learn more about Quality Engineering topics, visit: https://engineering.rently.com/quality-engineering/
Get to know about Rently at: https://use.rently.com/