Getting your Trinity Audio player ready...
|
This blog is all about how to connect EC2 with a single word using bash, take a look into it.
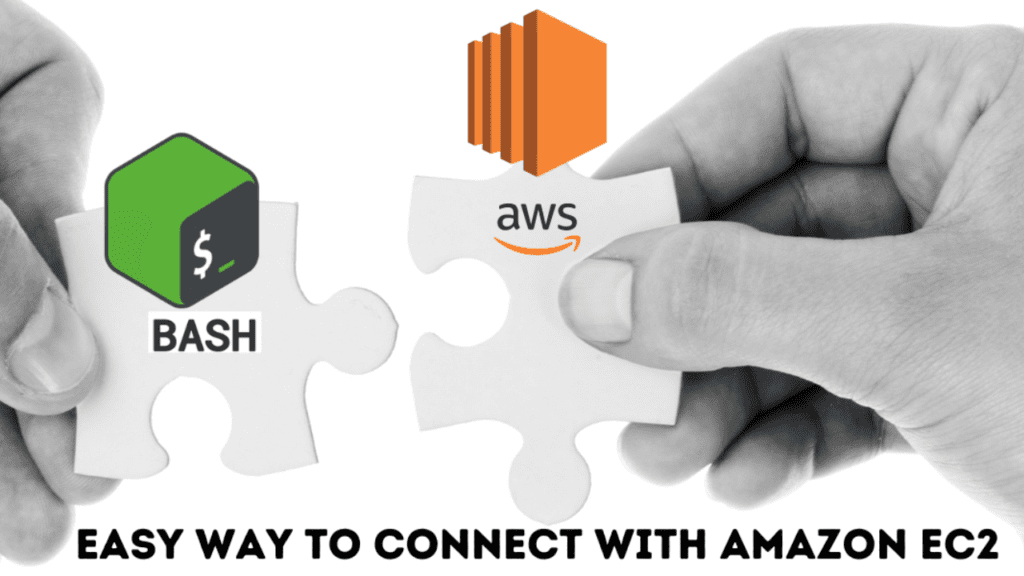
What is bash scripting?
“A Bash(or Bourne Again Shell) script is a plain text file which contains a series of commands. These commands are a mixture of commands we can normally type ourselves on the command line (such as ls or cp for example) in the linux terminals.”
Amazon EC2 Instance Connect
AWS EC2 is short for AWS Elastic Cloud Compute. EC2 is a virtual server in the AWS Cloud service.
One of the most common tools to connect to Linux servers by remote login is Secure Shell (SSH). It was created in 1995 and is now installed by default on almost every Linux distros.
When connecting to servers via SSH, SSH key pairs are often used to individually authorize users. As a result, the authorized user has to store, share, manage access for, and maintain these SSH keys.
Requirements to connect
- SSH Key( .pem file) provided by a particular Amazon EC2 instance. This SSH key is provided by Amazon when you launch a new instance.
- IP address assigned to your ec2 instance.
- The username depends on the Linux distro you just launched. Usually, these are the usernames for the most common distributions:
- Ubuntu: ubuntu
- Amazon Linux: ec2-user
- Centos: root
Connect to your EC2 Instance
Connect to an EC2 instance using SSH in Linux
- Launch the terminal and change directory with command cd, where you downloaded your pem file. In this demonstration, the pem file is stored in the VPN folder.
- Type the following SSH command based on your credentials
ssh -i file.pem username@host
The explanation of the above command
- ssh: Command to use SSH protocol
- -i: Flag that specifies to identify the key file.
- username: Username that uses your instance.
- Host: Public IP address given to your instance
- After executing the SSH command with your ssh key file, username and public ip address of your instance, a question will prompt to add the host to your known_hosts file. Type yes. This will help to recognize the host each time you’re trying to connect to your instance.
And that’s it! Now you’re logged in on your AWS EC2 instance
Bash script for EC2 instance connection with single word
In this we are using switch case statement in bash script.
Create a script file using the following command.
Using the above command, we create a shell file to store our bash script. The nano is a basic command-line text editor, but you can use whichever text editor you prefer(like vim, vi, mousepad, etc).
#! /bin/bash
In order for the computer to recognize our bash script and perform action, we must start the script file with a specific identifier called the declaration.
The declaration indicates the start of the bash script and to run the following commands. We do this with symbol sharp (#) and an exclamation point (!).
These characters(#!) together are all called “shebang”. Their only function is to title the absolute path to the bash interpreter. After the shebang, we have the interpreter, in this case its “/bin/bash”.
Below the shebang you’ll see the colored lines of command. In this we are using $1 in the switch case to pass a parameter. $1 is the first argument passed to the shell script by command-line. Also known as Positional parameters.
For example,
#./ec2connect vm
The ec2connect denotes the bash script filename and the vm denotes the parameter we are passing to execute the script. Below the shebang we use switch case statements to get the keyword of the instance to connect.
There are some key points of bash switch case statements:
- A case statement in bash scripting starts with the keyword ‘case’, followed by the case expression and ‘in’ keyword.
- The statement ends by the keyword ‘esac’.
- The ‘)‘ operator indicates the termination of a pattern list.
- The double semicolon (;;) used for termination of expression.
- An asterisk symbol (*) is used as a final pattern to define the default case.
After using the ssh command for a particular keyword like “vm”. If you have multiple AWS EC2 instances you can add more statements to connect whatever instance you want by keyword.Use CTRL + O and CTRL + X to overwrite and save the script file.
You can execute the script using 3 methods:
1. Using bash keyword to execute.
2. Using “./filename”
For executing in this method you need to add executable permission to the script file.
By using the above command you can add the executable permission.
3. Executing the file by adding to path.
To execute the script file you need to execute in the same directory where the script file is present or you need to specify the path, but we are able to execute them easily without specifying their absolute path or being in the directory. You can do this easily by moving the script file to /usr/local/bin directory. Make sure the path is added to your $PATH variable. To see what’s in your $PATH variable, type this into a terminal.
To see what’s in your $PATH variable, type this into a terminal.
You can add the script file wherever in the given path in command output.
Adding ec2connect file to path by using copy command:
After Adding the script file to the $PATH variable you can easily execute the file to connect to the EC2 instance.
Conclusion:
In this exercise, we have gone through bash scripting, EC2 instance, connecting through SSH, Switch case in bash and Three types of method used to execute script file.
References:
To learn more about this kind of blog, visit https://engineering.rently.com/quality-engineering/.