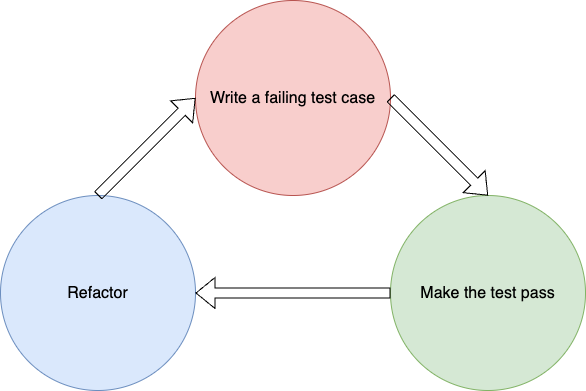
Test Driven Development (TDD) is software development approach in which test cases are developed to specify and validate what the code will do
Steps
1. Write a test case which will certainly fail
Before we even start writing our function we will first start with a very simple test case and run it to ensure that it fails. We ensure that the test fails first is to validate that the test case is written correctly. If we write the implementation first and later write the test cases then if the test passes we wont be sure if it’s passing because the implementation is correct or is it because the test case is written incorrectly. By writing and ensuring the test fails first we will avoid such confusion. This also ensures developer focus on the requirements before writing the code.
2. Make the test case pass
Write only enough code to make the test cases pass. The newly written test case and all existing test cases should pass at this step. The implementation need not be perfect as we will refactor and improve it in the next step. We have to ensure we don’t write any extra code than it is required to make the test cases pass.
3. Refactor
This is a good time to reflect and look at the full function and make improvements in the following areas.
- Code readability
- Remove duplication
- Follow coding guidelines
- Improve maintainability
During each change run the tests and confirm they are not breaking any existing features
- Lets the developer work on small subsets of requirements without having to worry about the overall impact.
- Helps the developer to try out multiple approaches while ensuring that requirements are still being met.
- Can confidently make last minute optimisations.
- Your test cases will ensure that any future changes to the function will not break your requirements.
- Helps free up developers mind by letting him focus first on requirements and then on implementation.
Example
Requirements for simple electricity bill generator program
- If units less than 200 then amount is calculated with the rate of 0.17$ per unit
- Program should accept consumed electricity units and return bill amount
- If units exceed 200 then units above 200 are calculated with the rate of 0.2$ per unit
- If units less than 30 then minimum bill is generated by treating consumed units as 50
Before even we start with the function lets write a basic test case which calls the function and checks the response
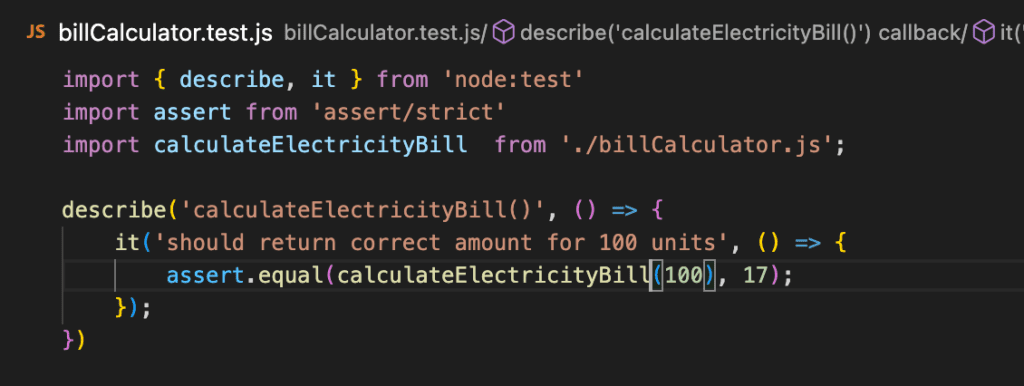
Of course this will fail
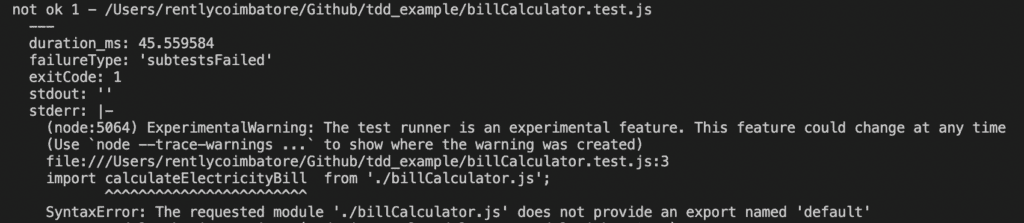
Now we should write only enough code to make this test pass
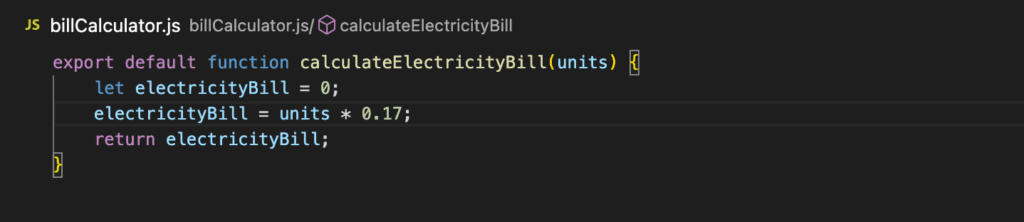
Lets check the requirements and add additional test cases
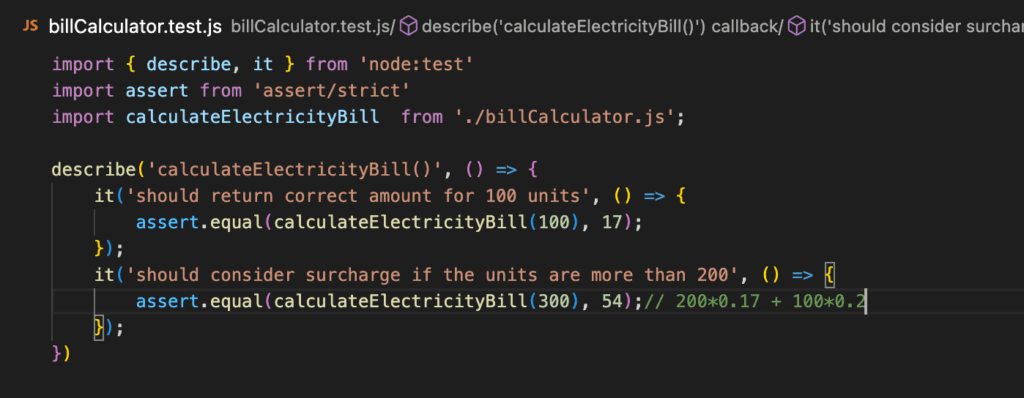
This should rightly fail
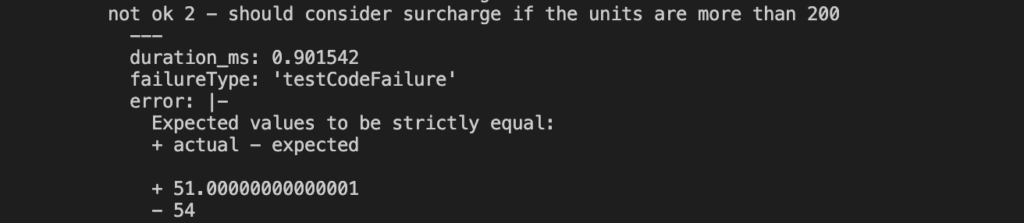
Lets handle this case in our function
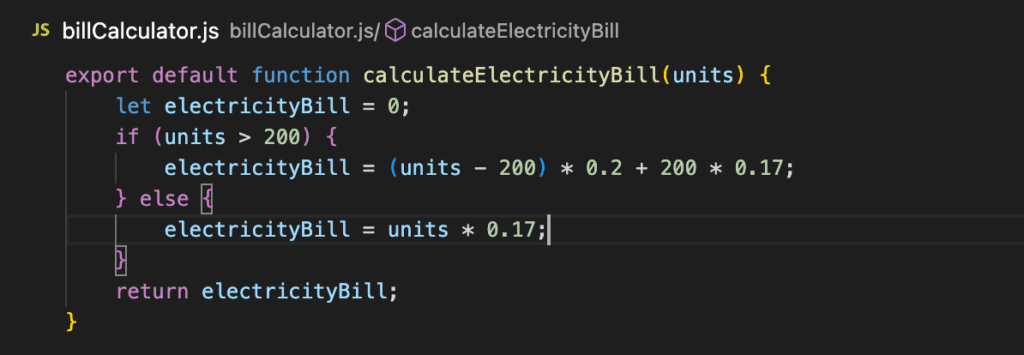
Only minimum bill case is pending now so lets add that test case as well
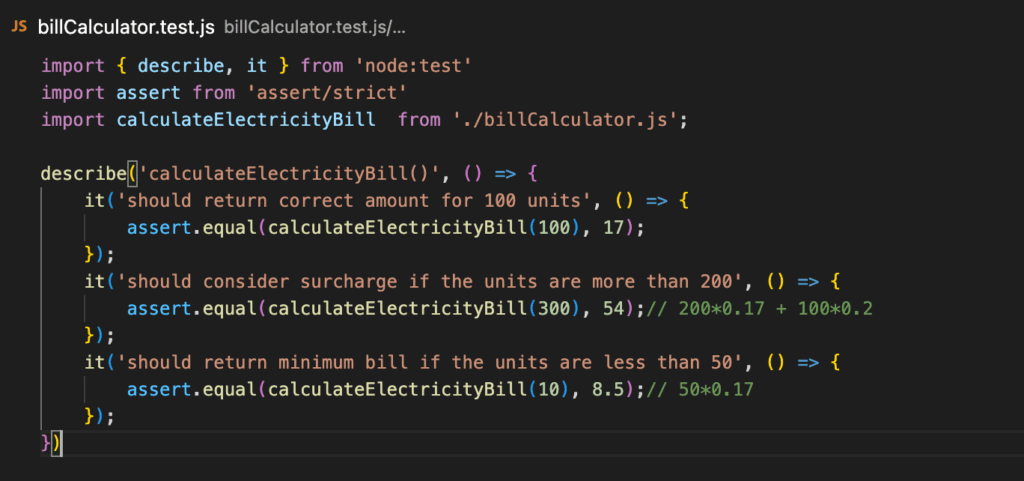
And we should make sure this test fails
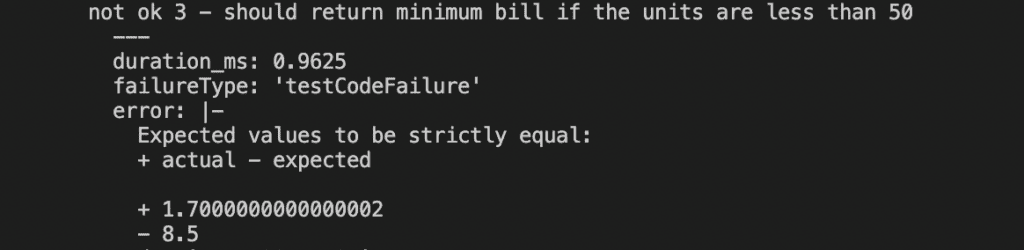
Now finally we will add this condition while making sure we are not breaking existing test cases
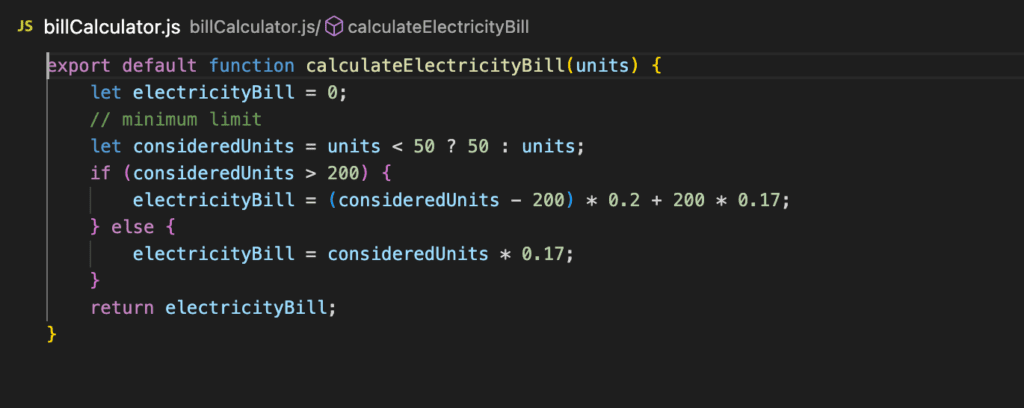
This completes our implementation and now would also be a good time to reflect on the implementation and make improvements in terms of readability and maintenance. After looking at the function the formulas look a bit complicated because we have directly used numbers. To improve readability we can use some variables.
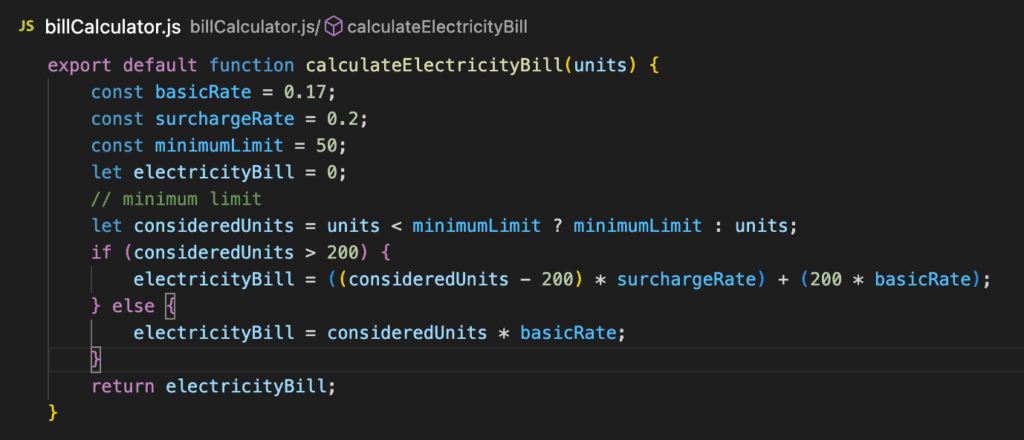
The test cases helped us make these last minute changes without worrying about breaking the requirements.
References:
https://nodejs.org/api/test.html
https://en.wikipedia.org/wiki/Test-driven_development
To learn more about Engineering topics visit – https://engineering.rently.com
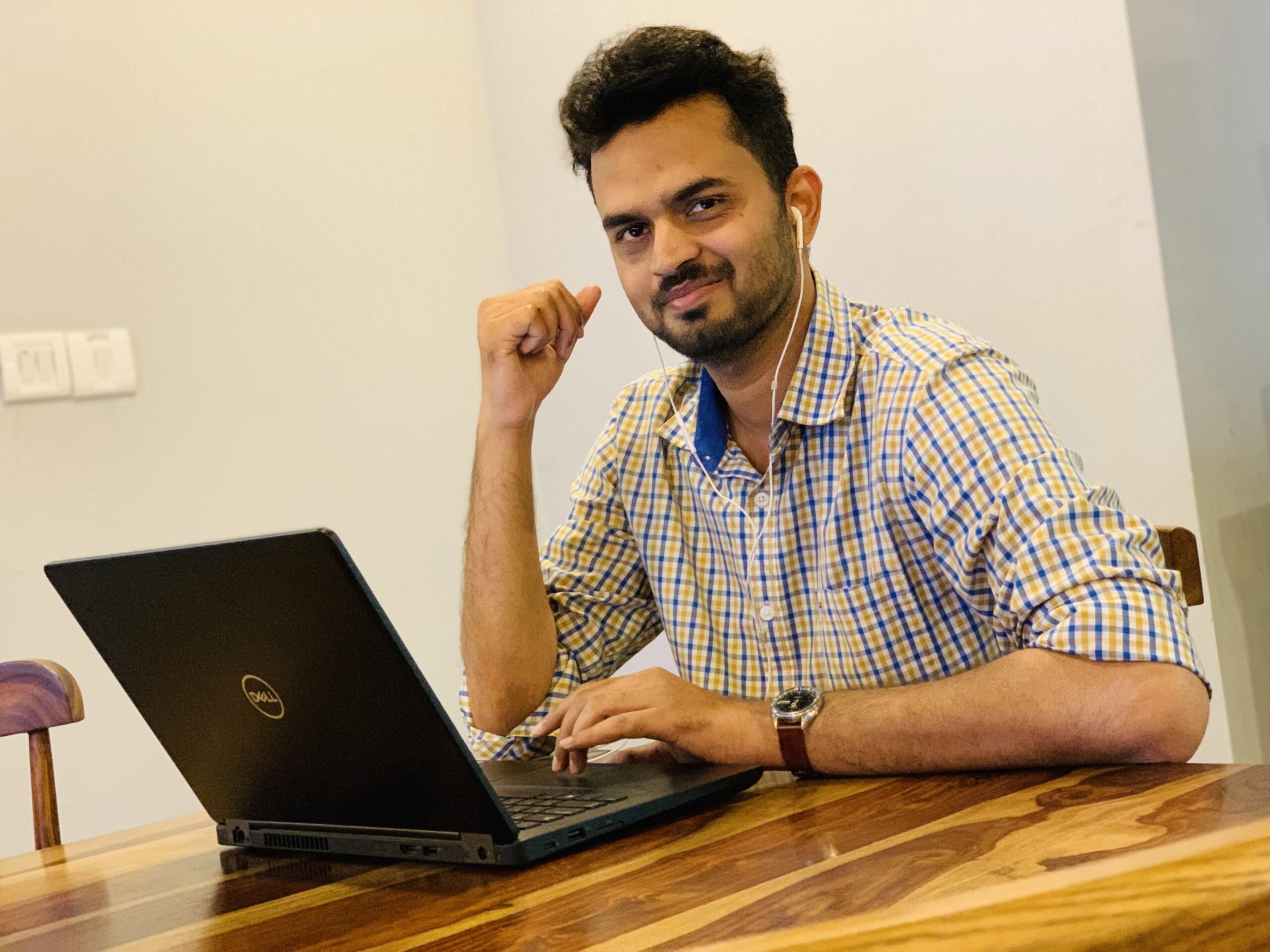
Node.js, Serverless
Good document it is!