Introduction
Ruby is celebrated for its expressiveness and elegance. Much of its charm stems from the concept of metaprogramming. In this blog, we delve into the captivating realm of Ruby metaprogramming, uncovering its historical origins and emphasizing its advanced features that set it apart from other programming languages.
What is Metaprogramming?
Metaprogramming involves crafting code that generates more code. This concept is not new; it has been part of programming since the early days of assembly language. However, modern languages like Ruby and Python have elevated metaprogramming to new heights.
Why Metaprogramming?
- Dynamic Coding: Metaprogramming leverages the power of dynamic coding, enabling developers to adapt their software swiftly to changing requirements.
- Flexibility: It provides unparalleled flexibility, empowering developers to craft code that adapts seamlessly to diverse situations and scenarios.
- Eliminating Duplication: Metaprogramming excels in eradicating code redundancy by constructing universal patterns and behaviors that find utility throughout an application.
- Customization: It empowers developers to customize the functionality of objects, tailoring their behavior to meet specific needs.
Elements of Metaprograming
Ruby encompasses several essential elements that facilitate metaprogramming:
- send
- define_method
- class_eval and instance_eval
- method_missing
Implementation of send method
The send method in Ruby is a versatile tool, allowing developers to invoke methods dynamically.
Used to assign the property value for a object in runtime
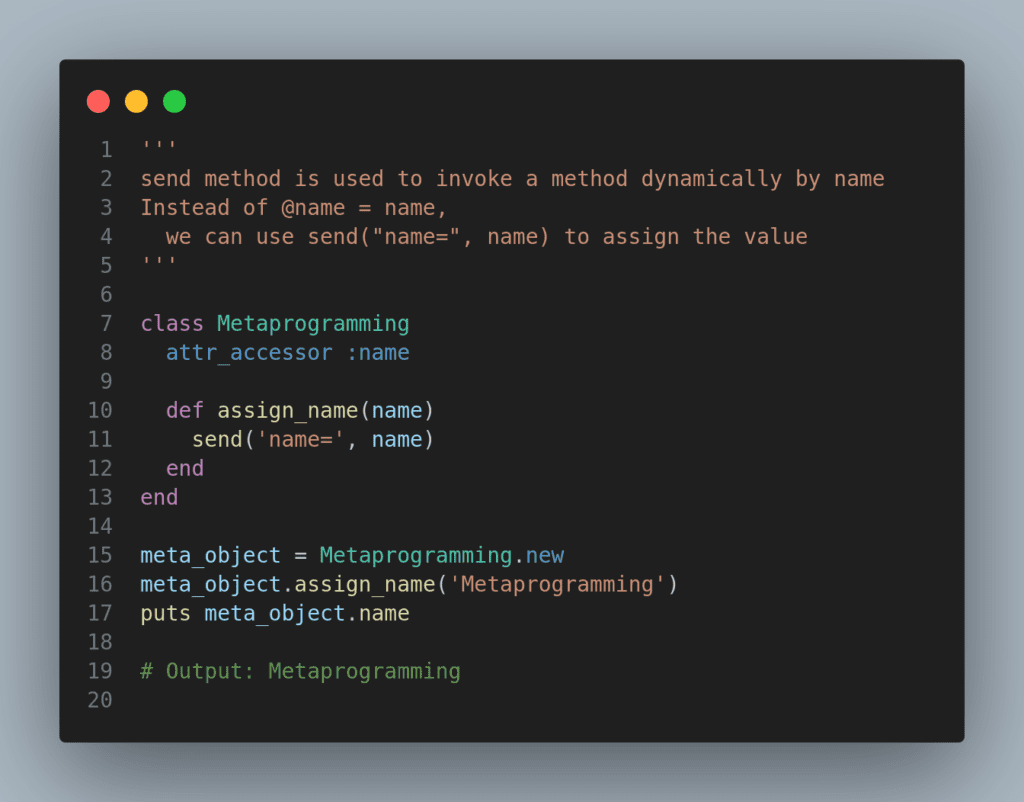
In Metaprogramming Example 1.1, an attribute accessor named ‘name’ is present within a Metaprogramming class. During object initialization, this attribute accessor isn’t assigned a value. Instead, the value is dynamically assigned when ‘meta_object.assign_name(‘Metaprogramming’)’ is invoked, showcasing the ability to set property values during runtime, a core aspect of metaprogramming.
Used to call a method dynamically in runtime
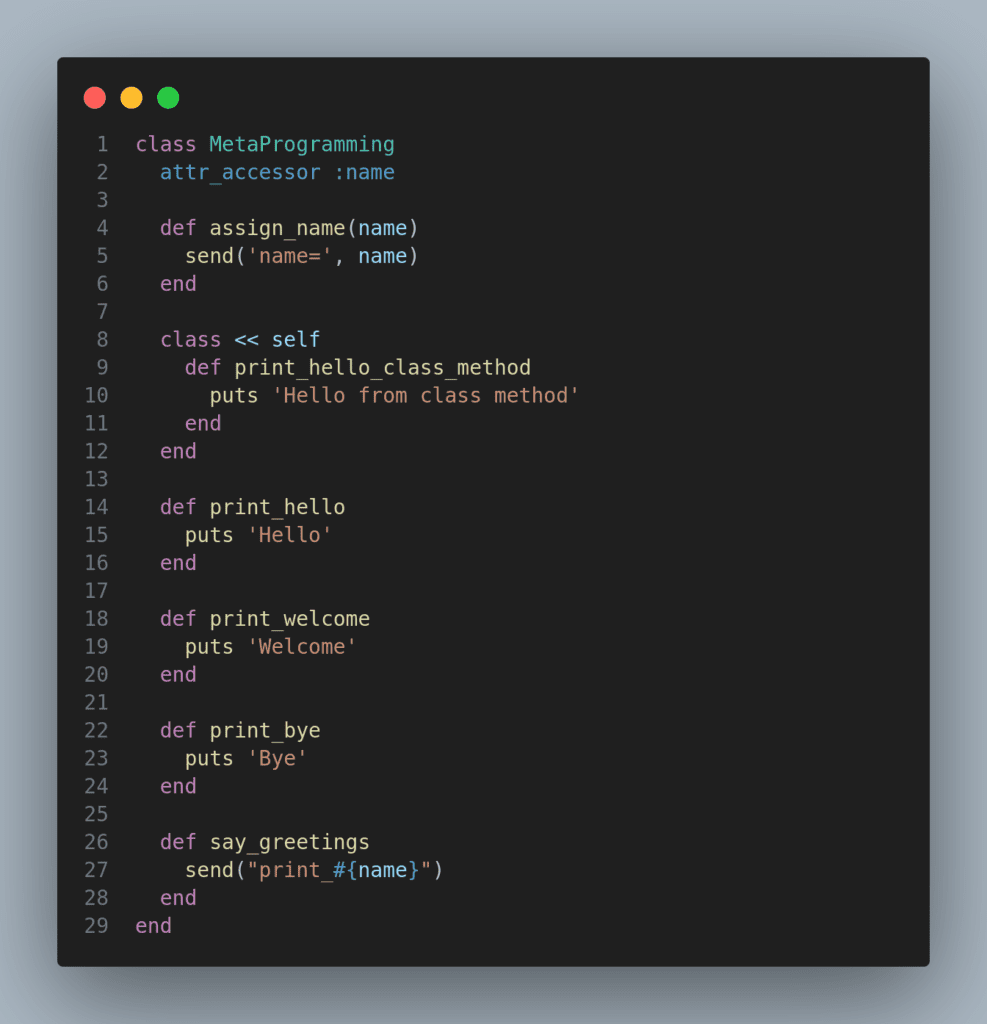
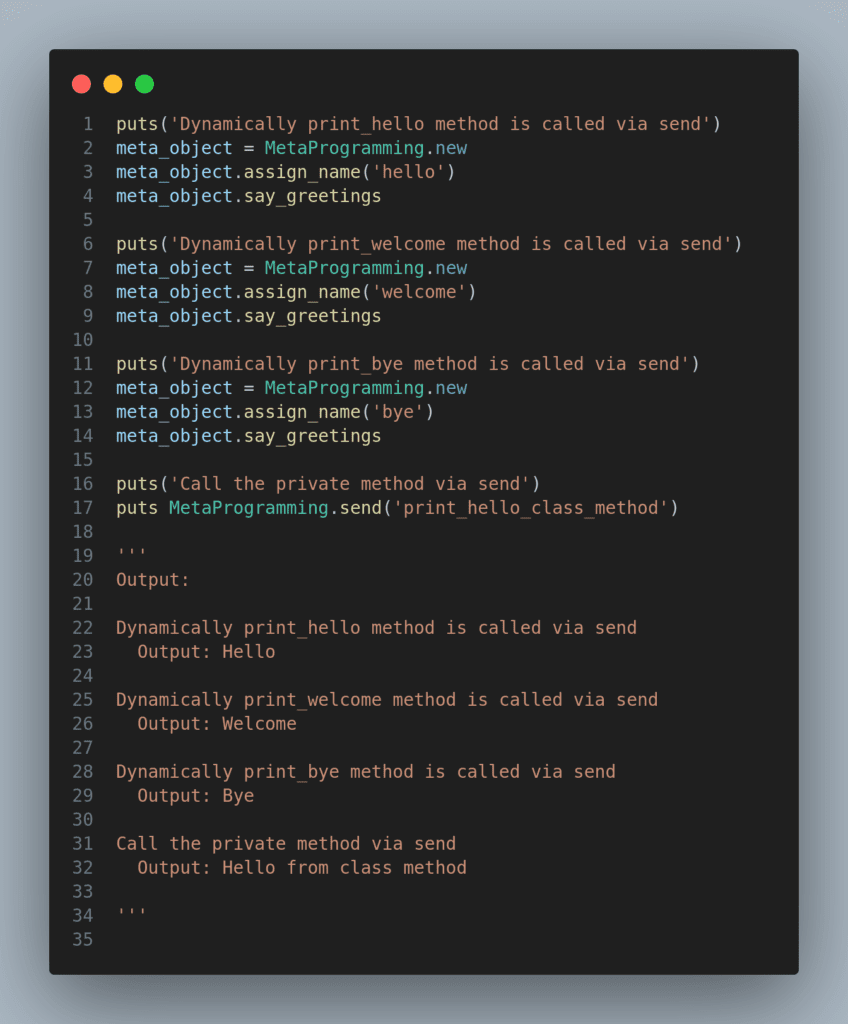
In Metaprogramming Example 1.2.x, a set of methods named ‘print_hello,’ ‘print_welcome,’ and ‘print_bye’ can be found. These method names follow a common pattern, which is ‘print_.’ By examining the code implementation, it becomes evident that the method to call is determined based on the value of the attr_accessor. Without employing the metaprogramming concept, calling these methods would necessitate the use of conditional statements, as demonstrated in Metaprogramming Example 1.3.
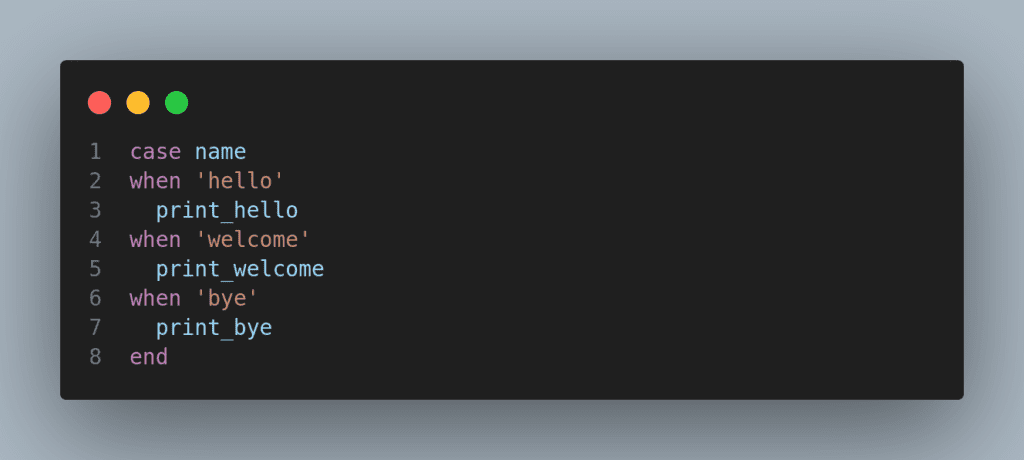
Using the ‘send’ method, code length can be significantly reduced. It allows dynamic method invocation, enabling the calling of both instance and private methods, a practice that streamlines the code and enhances its efficiency.
Used to create a method in association with define_method
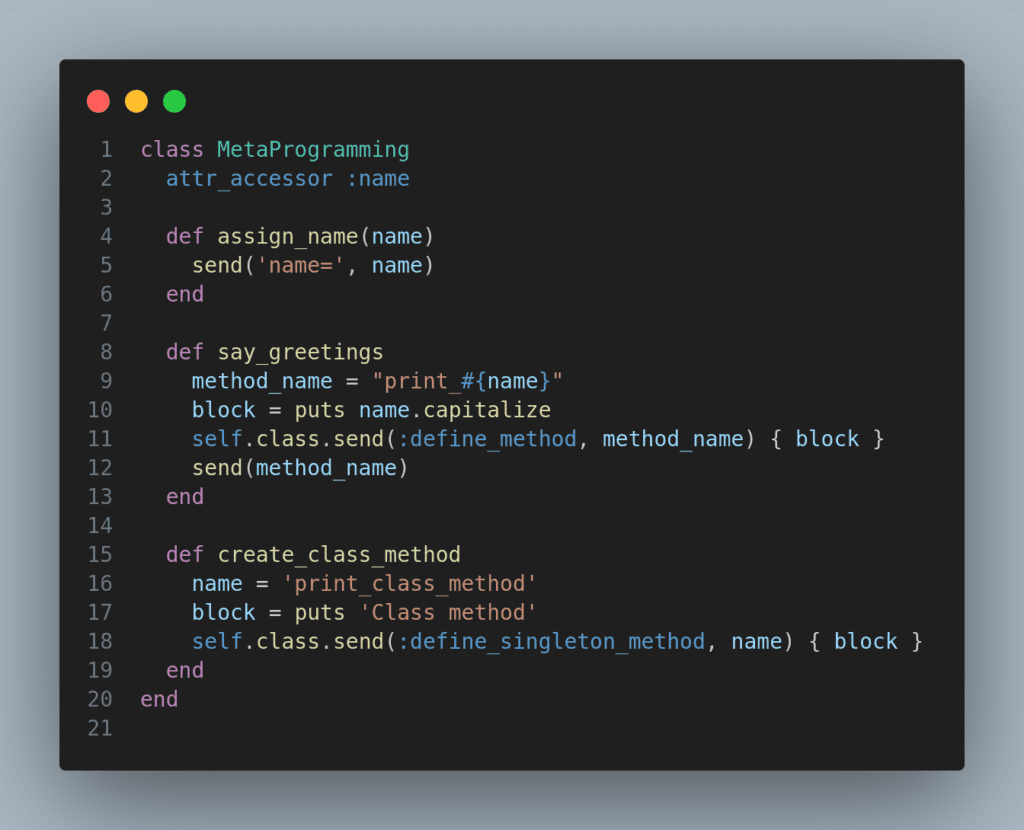
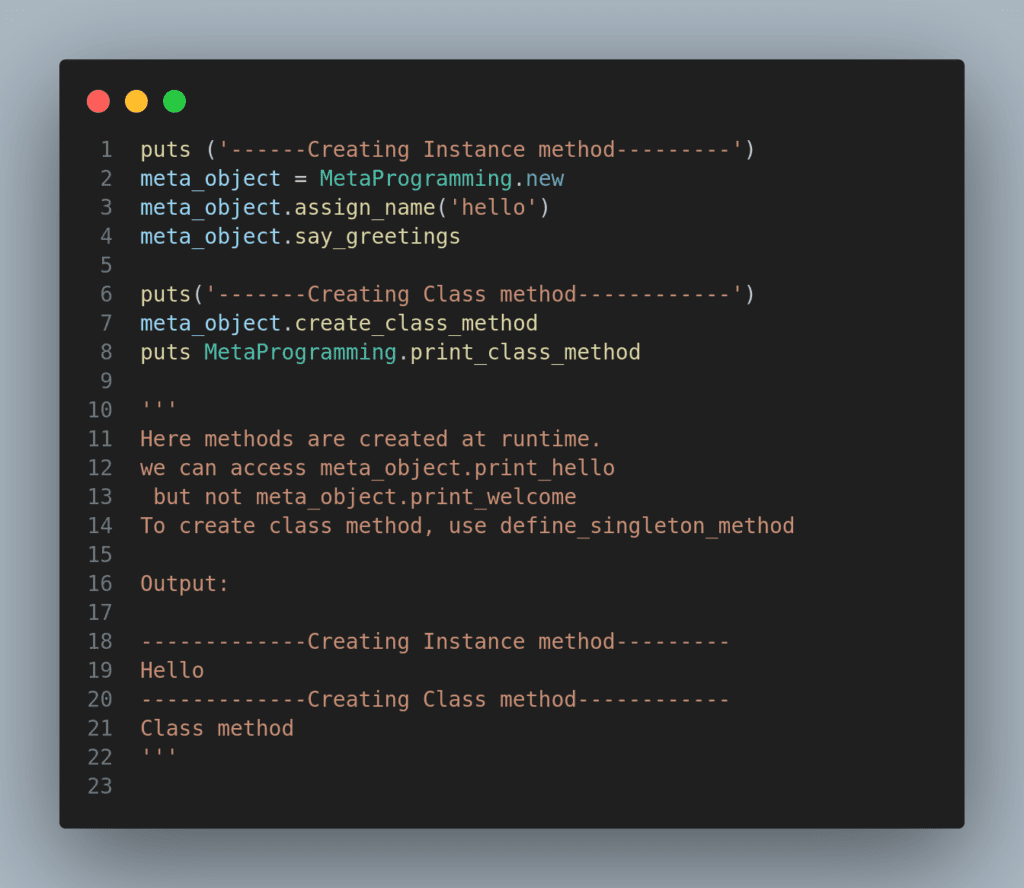
The ‘send’ method can also be used along with another metaprogramming element ‘define_method.’ ‘define_method’ is used to define methods in runtime. More explanation will be provided while explaining the ‘define_method’ element. In Metaprogramming Example 1.2.x, three different methods (‘print_hello,’ ‘print_welcome,’ and ‘print_bye’) are defined separately and called dynamically. But with the help of ‘send,’ we can create instance and class methods dynamically by passing ‘define_method,’ method name, and a block of code as arguments, as shown in Metaprogramming Example 1.4.x. It reduces the code length even more!
To create class methods, we should use ‘define_singleton_class.’
Using ‘public_send’ for Better Encapsulation:
While ‘send’ enables the invocation of both public and private methods of a class from any other class, it raises concerns about breaking the encapsulation property of Ruby. Don’t worry! With the usage of ‘public_send,’ we can overcome this, as shown in Metaprogramming Example 1.5.x.
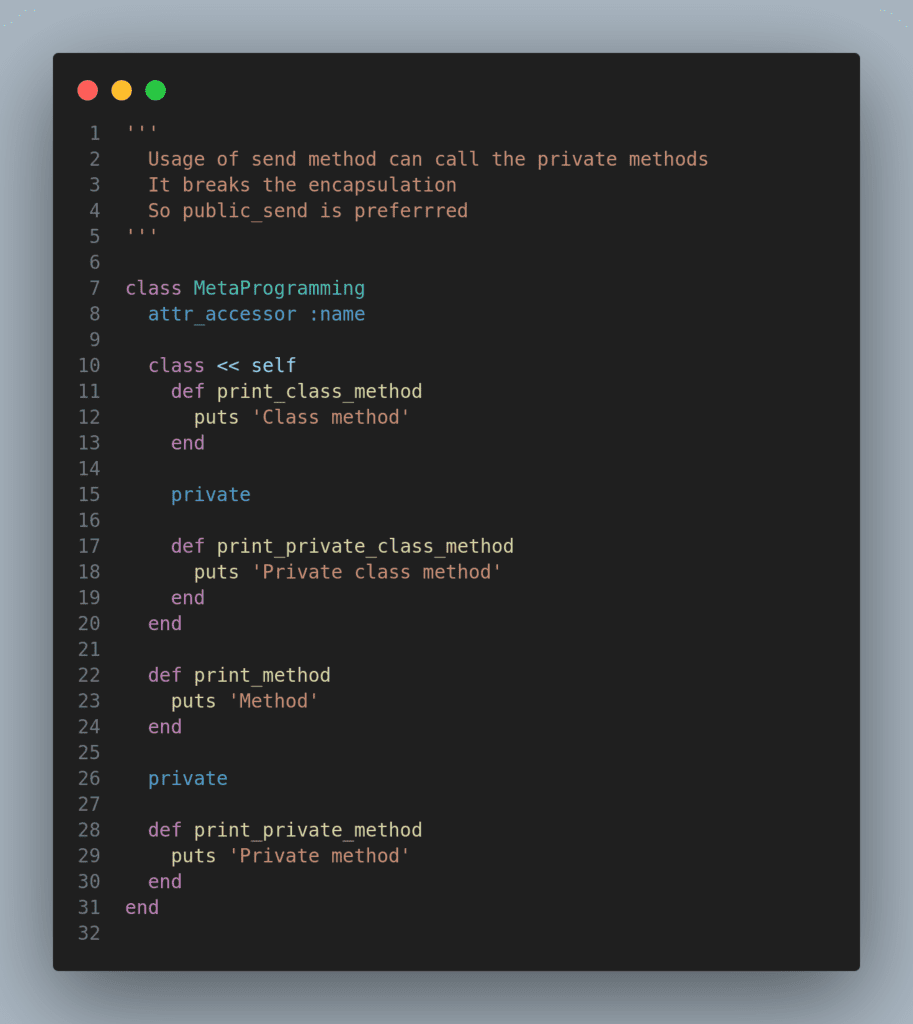
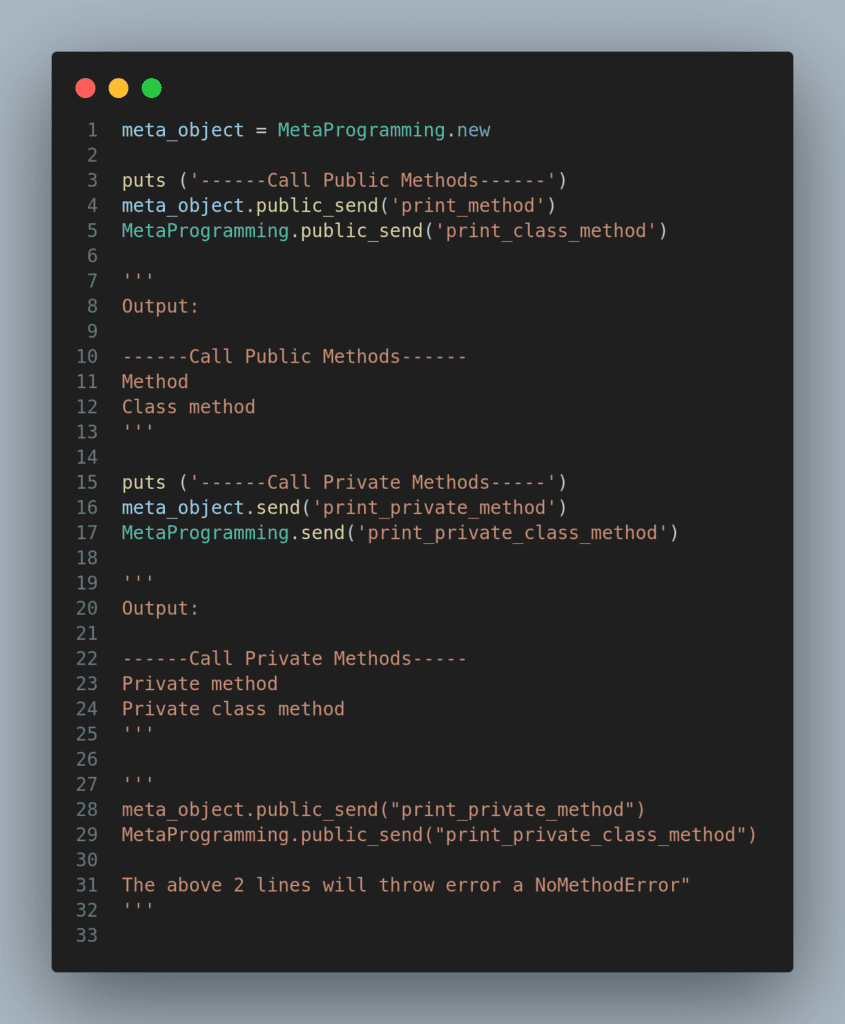
Implementation of define_method
As already discussed, with ‘define_method,’ you can create methods dynamically. This can be done without the ‘send’ method as well. To define class methods, ‘define_singleton_class’ should be used. Let’s see how it is done via Metaprogramming Example 2.1.
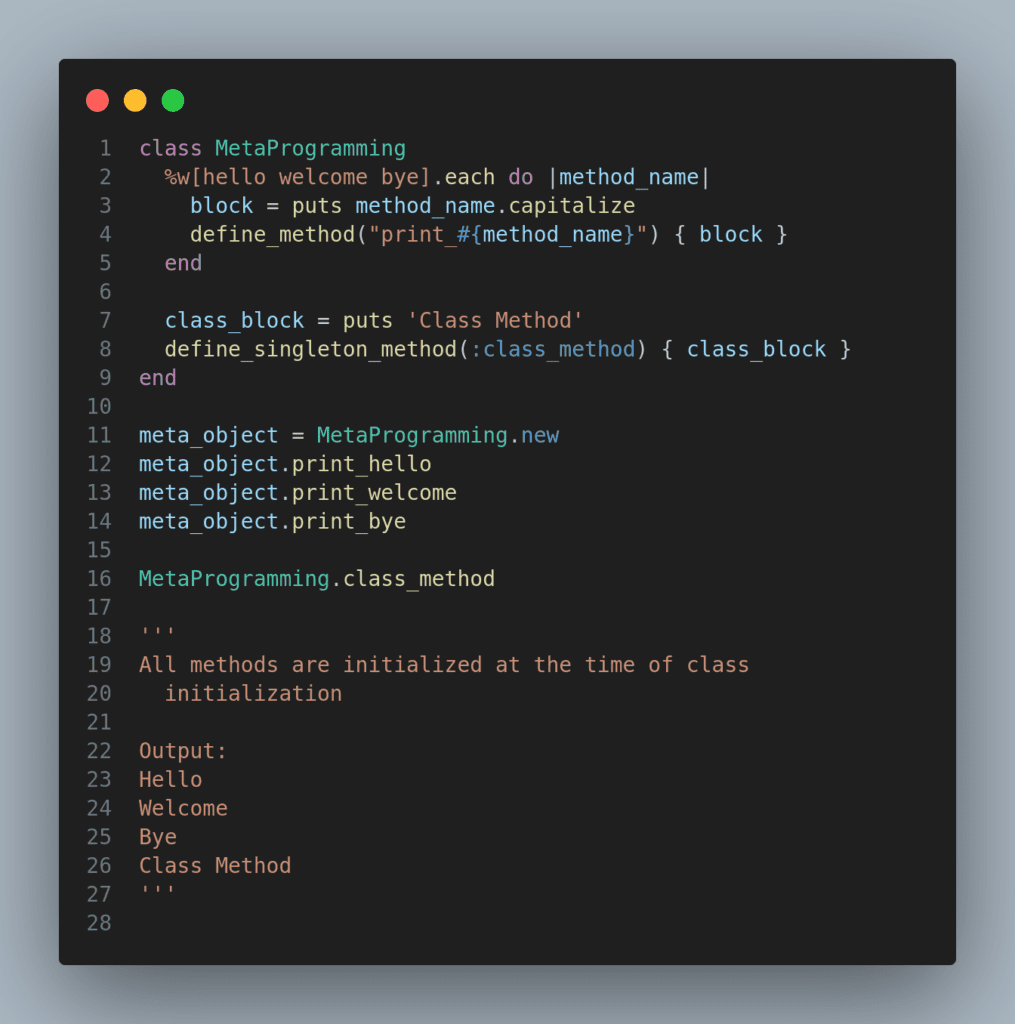
It’s cool right!!! Still there are 2 elements. Come on lets check that as well…
Implementation of class_eval and instance_eval
‘Class_eval’ and ‘instance_eval’ serve the purpose of defining and modifying the behavior of methods. This is achieved by utilizing the concept of Open class and MonkeyPatching, where the class is opened to perform the necessary modifications.
Using ‘class_eval’
‘Class_eval’ allows the execution of a block of code within the context of a class, enabling the creation or modification of method behavior at runtime (Applicable to all instances of a class)
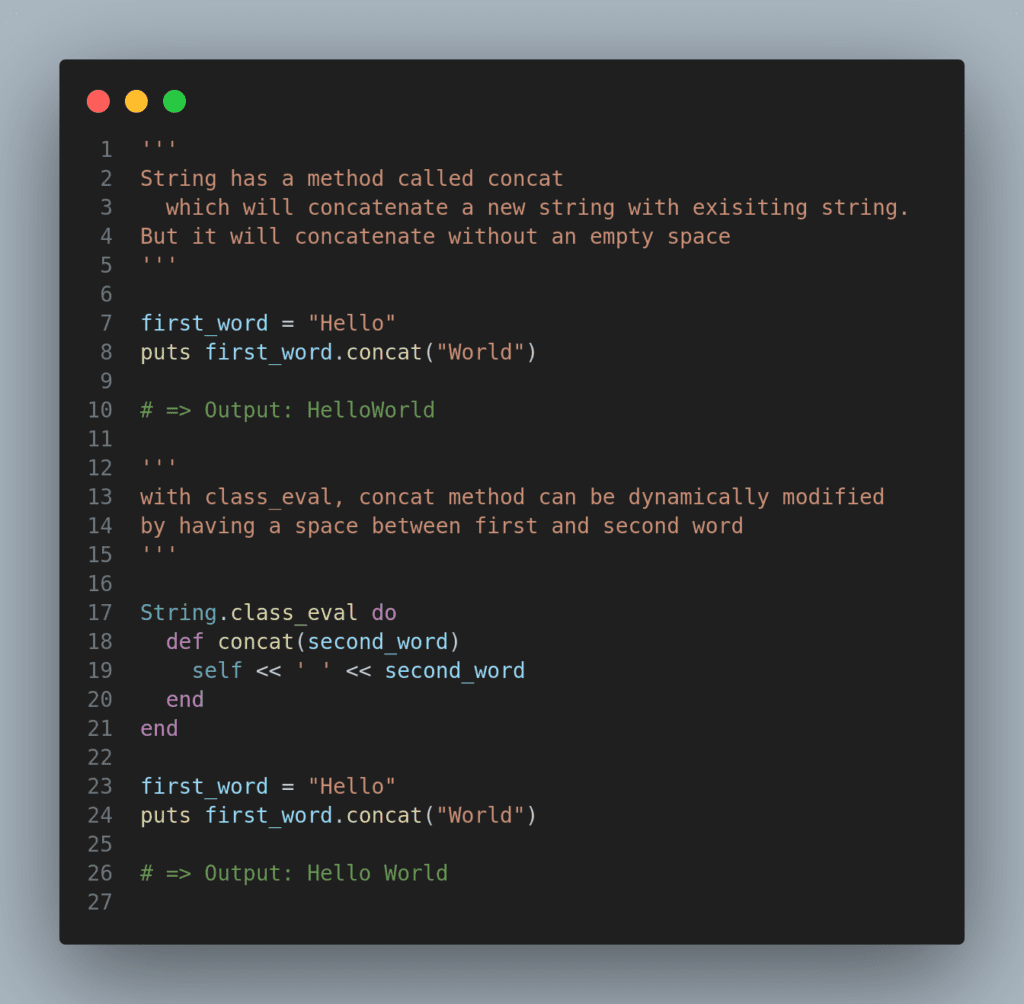
For instance, in Metaprogramming Example 3.1, the ‘String’ class’s ‘concat’ method is modified to merge strings with a white space between them. This modification applies to all instances of the ‘String’ class.
Using ‘instance_eval’
‘Instance_eval’ permits the execution of code within the context of a specific instance of a class, enabling the creation or modification of method behavior for that specific instance, in contrast to ‘class_eval,’ which affects all instances of a class.
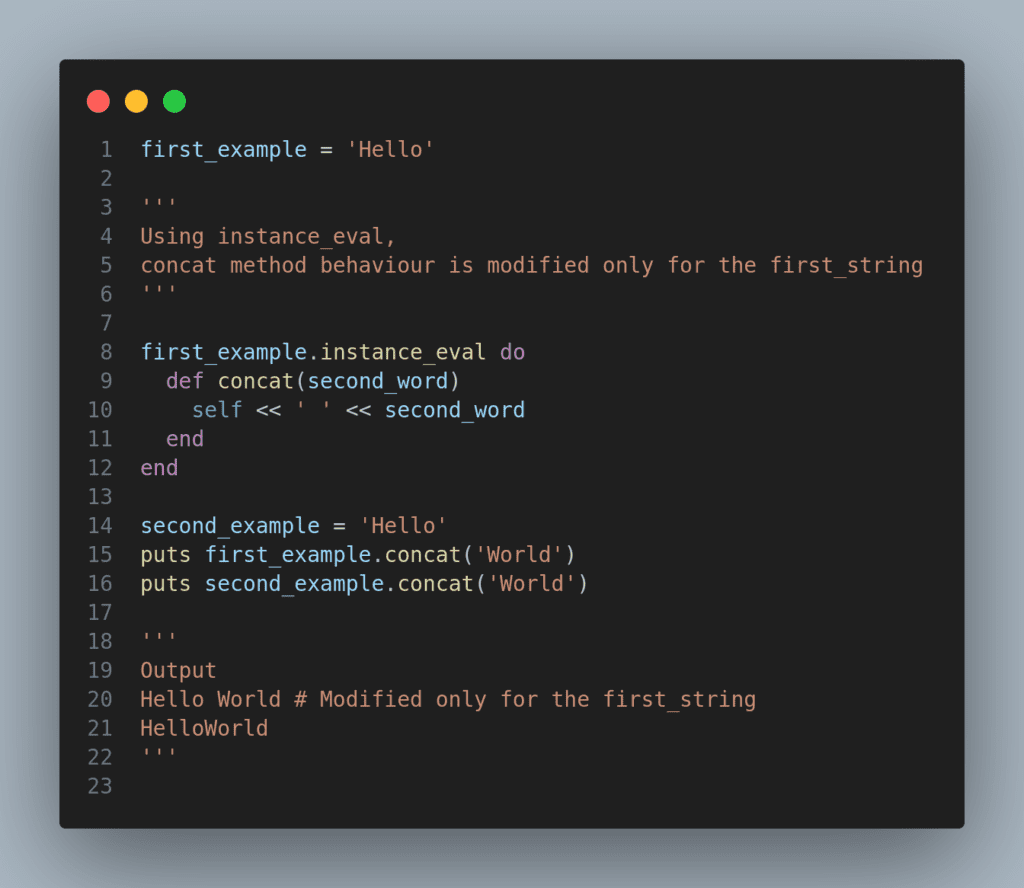
In Metaprogramming Example 3.2, ‘instance_eval’ is applied to a specific string. As a result, the modification is exclusively applied to ‘first_example’ and does not affect ‘second_example.’
Dynamic Method Definition Using class_eval and instance_eval in Metaprogramming
These techniques allow developers to define methods, and the accessibility of these methods depends on which ‘eval’ method is used. For instance, consider an instance variable, ‘@name,’ and the need for a method named ‘name’ to retrieve the value of this instance variable.
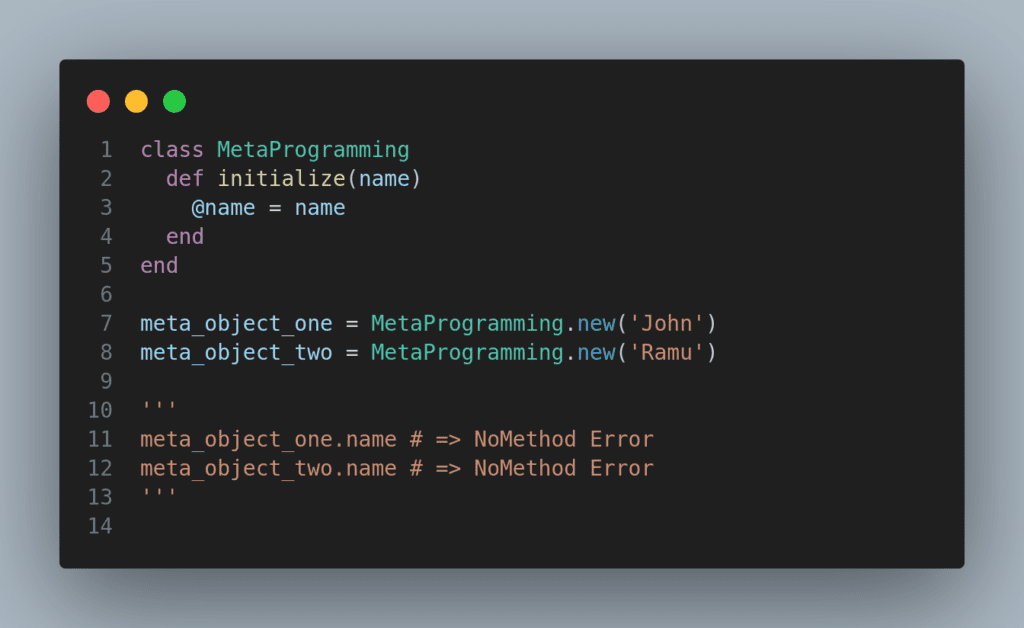
In Metaprogramming Example 3.3, there is no ‘name’ method initially. Attempting to access ‘name’ results in an error.
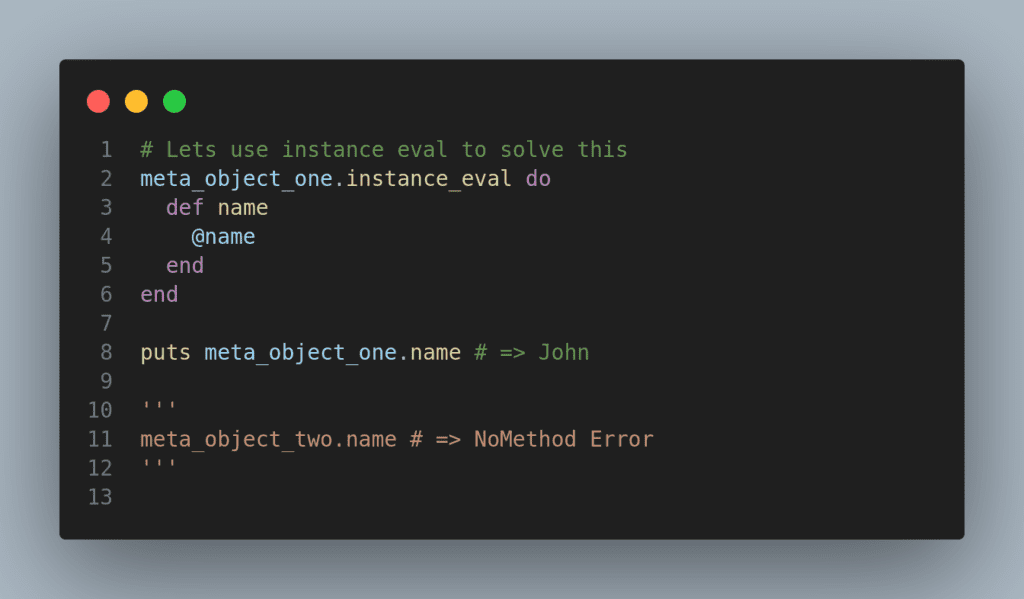
In Metaprogramming Example 3.4, ‘instance_eval’ is used to define a method for ‘meta_object_one.’ However, ‘name’ can only be accessed on ‘meta_object_one’ and not on ‘meta_object_two.
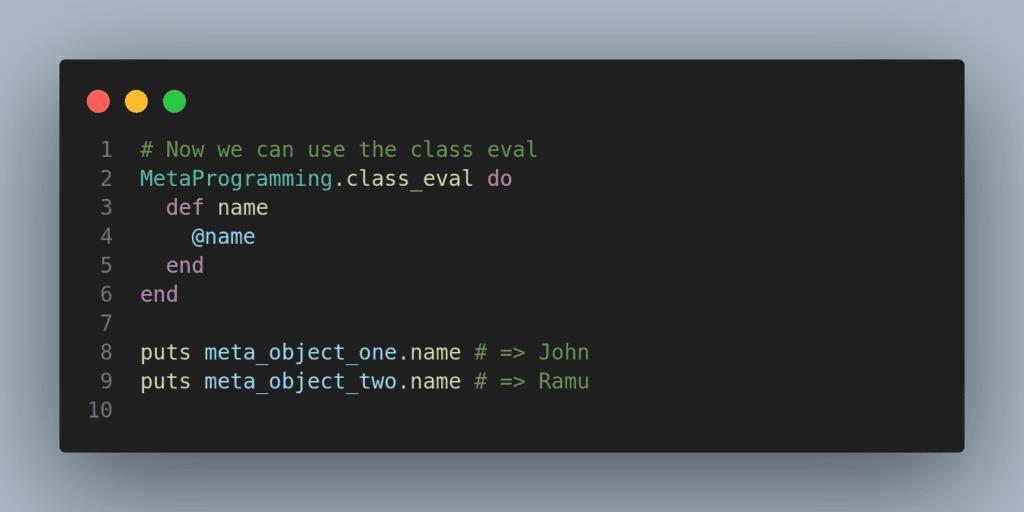
Metaprogramming Example 3.5 demonstrates the use of ‘class_eval’ to define the method. With this approach, the ‘name’ method can be accessed by all instances of that class.
Implementation of method_missing
This is one of the intriguing elements of metaprogramming that makes Ruby even more expressive. When a method is not defined in a class and an attempt is made to access that method, Ruby raises a NoMethodError. This behavior is handled by Ruby via ‘method_missing.’
Understanding method_missing
‘method_missing’ is an inbuilt method that gets called when a method is not defined on a class, resulting in an error. However, through the application of metaprogramming, we can dynamically alter the behavior of this method.
‘method_missing’ takes three parameters: the name of the method, the arguments of that method, and the block passed to that method.
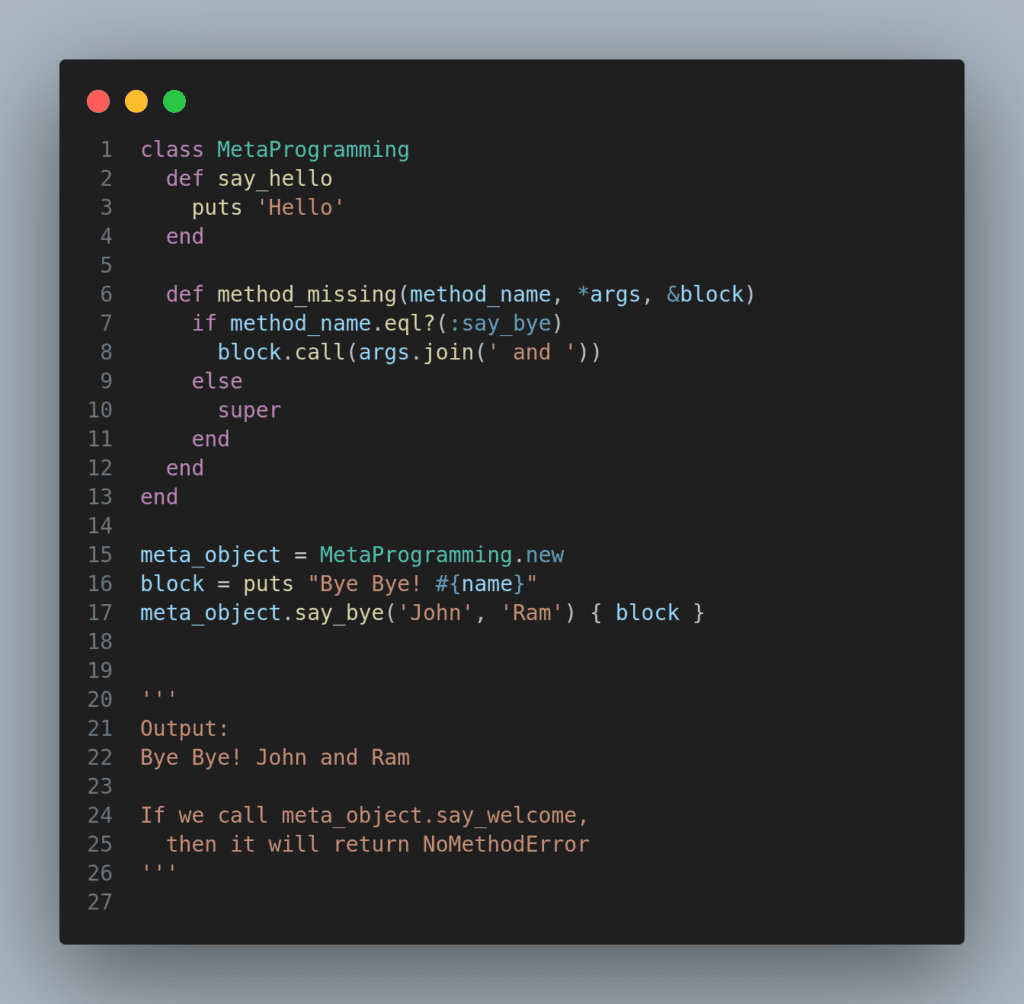
Custom Behavior with method_missing
In Metaprogramming Example 4.1, we open the ‘method_missing’ method and alter its behavior for a ‘say_bye’ method. The ‘say_bye’ method is called with two parameters and a block to print the needed message. For other methods that are not defined, it will still throw an error.
The Challenge of ‘respond_to?’
But, there’s a twist 😅
In Ruby, you can check whether a method is defined for a class or not using ‘respond_to?’ If you check the ‘say_bye’ method with ‘respond_to?’, it will return false, as demonstrated in Metaprogramming Example 4.2.
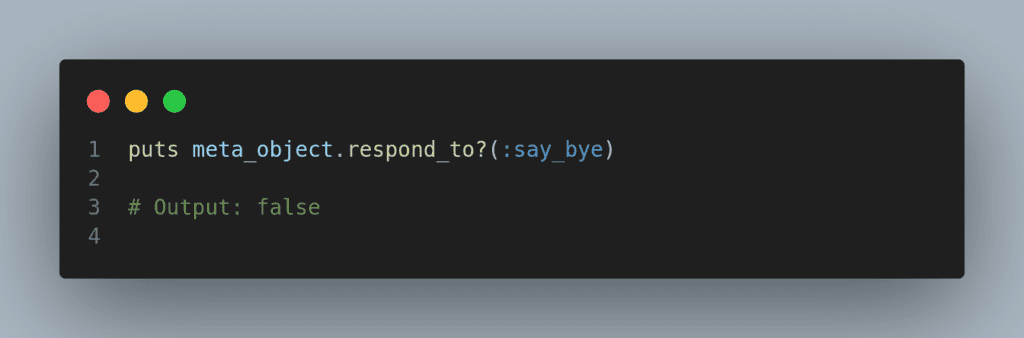
Addressing ‘respond_to?’ Challenges
Surprising, isn’t it? 😱
Yes, to avoid this, it is recommended to adjust the ‘respond_to_missing?’ method when changing the ‘method_missing’ behavior, as shown in Metaprogramming Example 4.3.
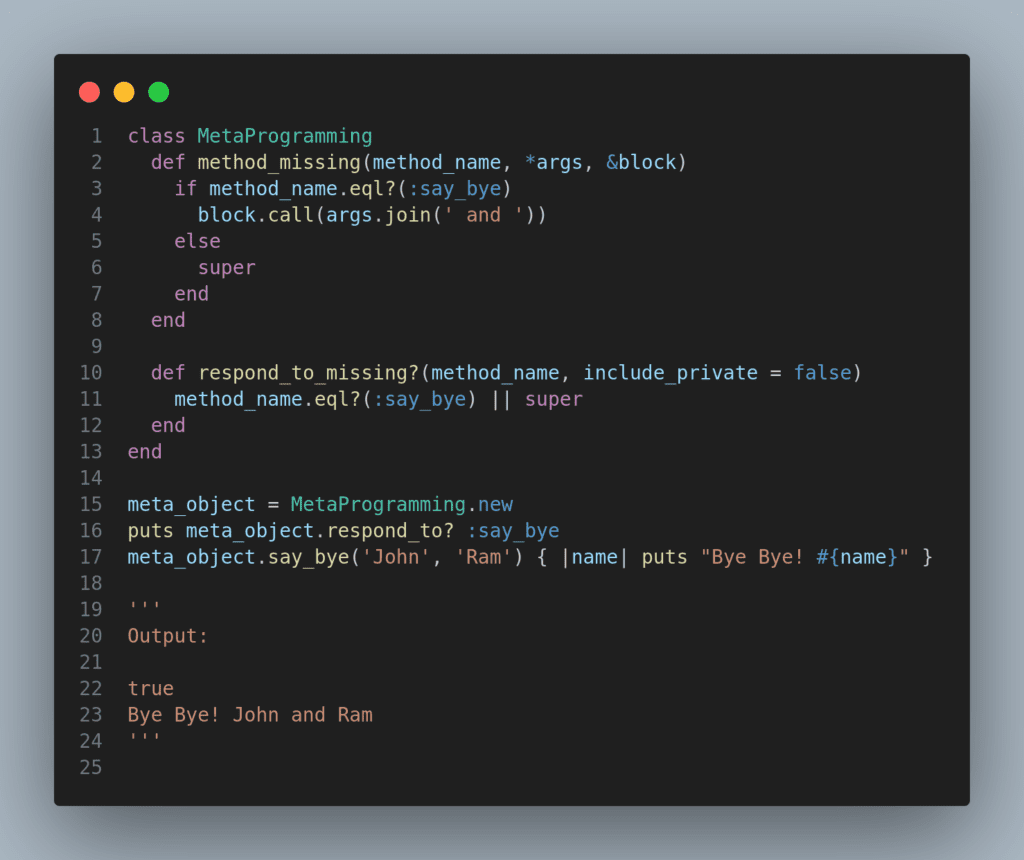
‘respond_to_missing?’ vs. ‘respond_to?’
Some older articles might recommend altering ‘respond_to?’ instead of ‘respond_to_missing?’
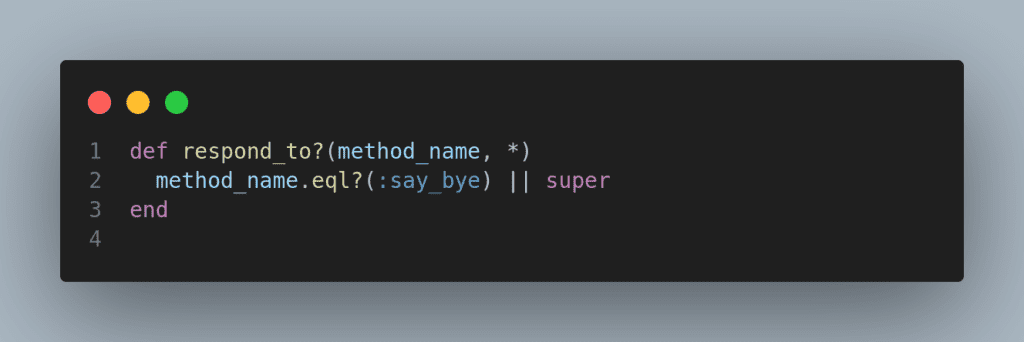
Ensuring Proper Functionality
Metaprogramming Example 4.4 will return true for ‘meta_object.respond_to?(:say_bye).’ But it can lead to trouble when a method is called indirectly.
Indirect Method Calls
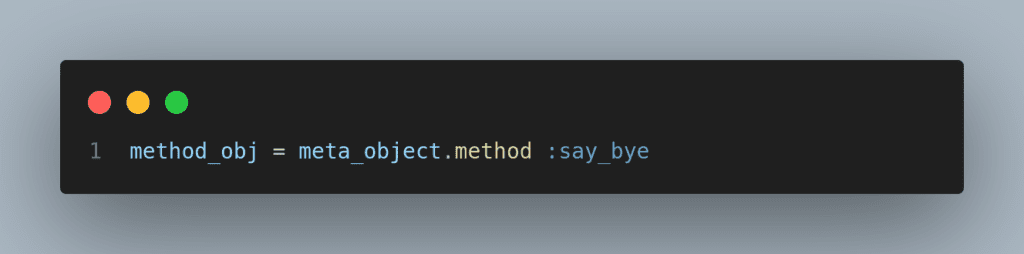
What does it mean to call a method indirectly? In Metaprogramming Example 4.5, a method object is created instead of calling the method directly. With this method, we can call the method with arguments and blocks. However, if you alter ‘respond_to?’ instead of ‘respond_to_missing?’, it will throw an error, as shown in Metaprogramming Example 4.6.
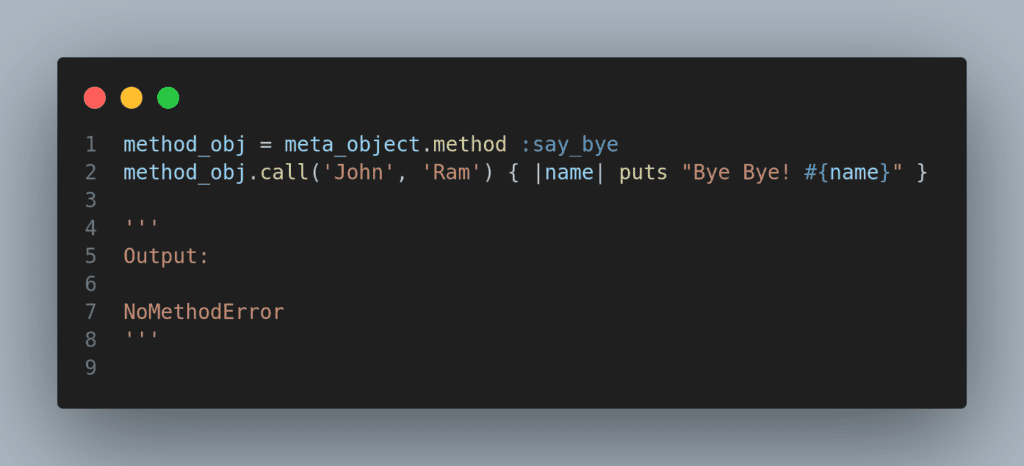
Enhancing ‘respond_to_missing?’
If the ‘respond_to_missing?’ method is altered, then indirect method calls will also work correctly, as shown in Metaprogramming Example 4.7.
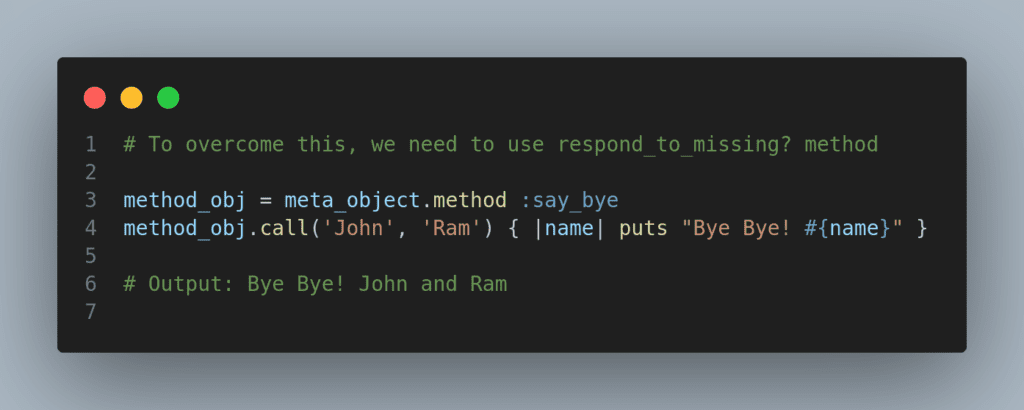
Alright, we have discussed about all the 4 elements of metaprogramming in ruby.
Caveats and Best Practices
- Complexity: Metaprogramming can increase code complexity and impact readability. Proper documentation and comprehensive test cases can mitigate this challenge.
- Performance: Depending on usage, metaprogramming can lead to performance degradation as operations are performed at runtime. It’s essential to monitor and optimize where necessary.
- Security: The flexibility to override behaviors can lead to potential security issues if not used judiciously.
Conclusion
Ruby metaprogramming is a fascinating and powerful aspect of the language that empowers developers to create dynamic and flexible code. By understanding and using these metaprogramming techniques judiciously, developers can harness the full potential of Ruby, crafting elegant and adaptable solutions to meet evolving software challenges. While it comes with challenges, the rewards of metaprogramming in Ruby are well worth the journey.
Reference
[1] Make you hands dirty by working with examples
[3] class_eval and instance_eval