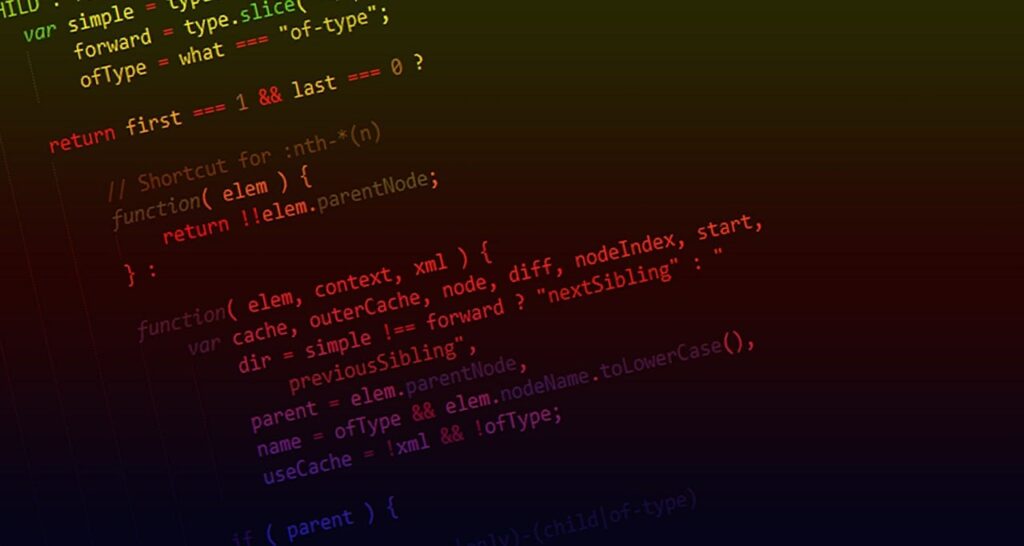
Have you ever faced a situation where your state
defined in the react component is empty or not working as expected, no matter how many times you update it? Or Your Function doesn’t return what you expected? If you did, then you might have faced a common pitfall named the Stale Closure Problem.
The Problem
Let’s understand from a simple example. Consider this seemingly innocent React component that has a form which sends the value provided in the SearchBar
to the backend. At first glance, everything appears to be in order:
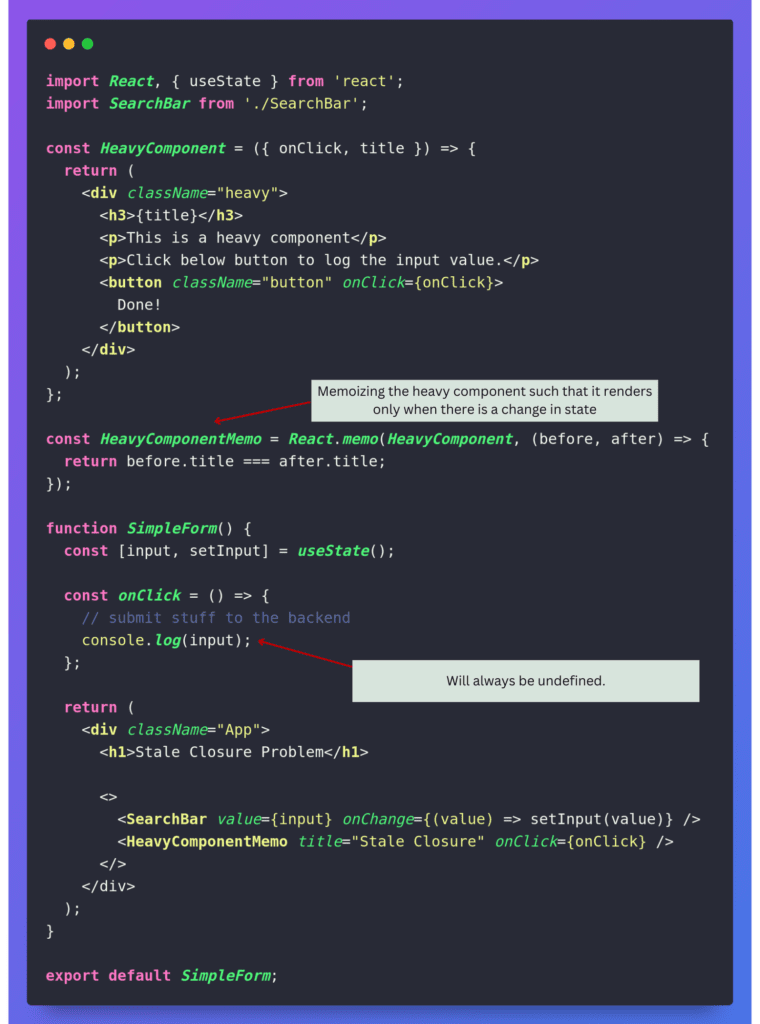
Exploring the Example:
The above code will generate a basic UI form
. The goal for the component is to log the input
value entered in the SearchBar
component when the Done!
button is clicked.
Unfortunately, it doesn’t behave as intended. Whenever the Done!
button is clicked, the printed value
will consistently be undefined
.
This issue is known as the Stale Closure Problem.
If you’re interested in witnessing the issue firsthand, take a look at the codesandbox link provided here.
Closure
Before we delve into the intricacies, let’s establish a basic understanding of closures. A closure allows a function to access a variable on an enclosing scope or an environment even after it leaves the declared scope.
When we create a function in JavaScript, we create a scope. The value inside the function is accessible only within the scope, not outside. Whereas the inner scope can access variables from the outer scope.
This happens whenever we create a function.
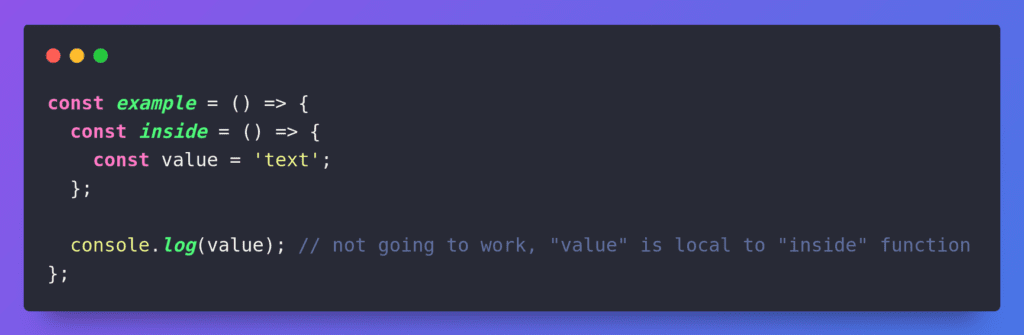
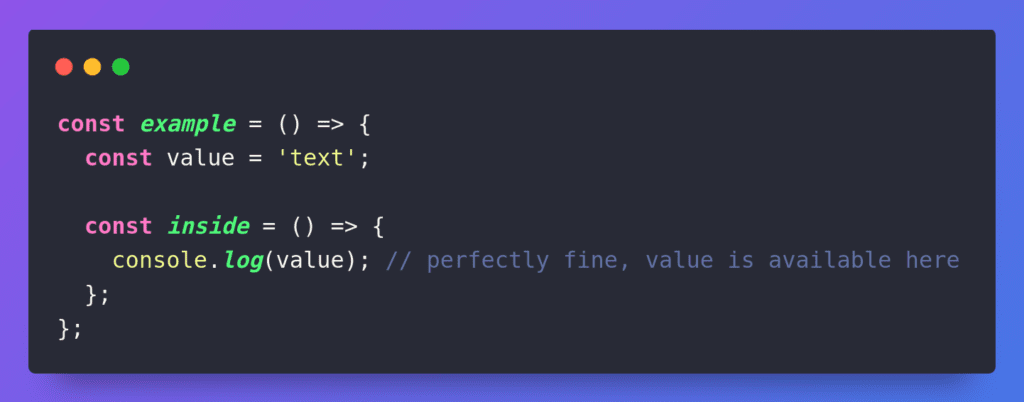
We call this behaviour “Closure”. The function inside takes a snapshot of all the variables and values surrounding the function and stores it in the memory.
Instead of creating a variable inside the function, we pass it in an argument and return the inside
function. We get the below behavior.
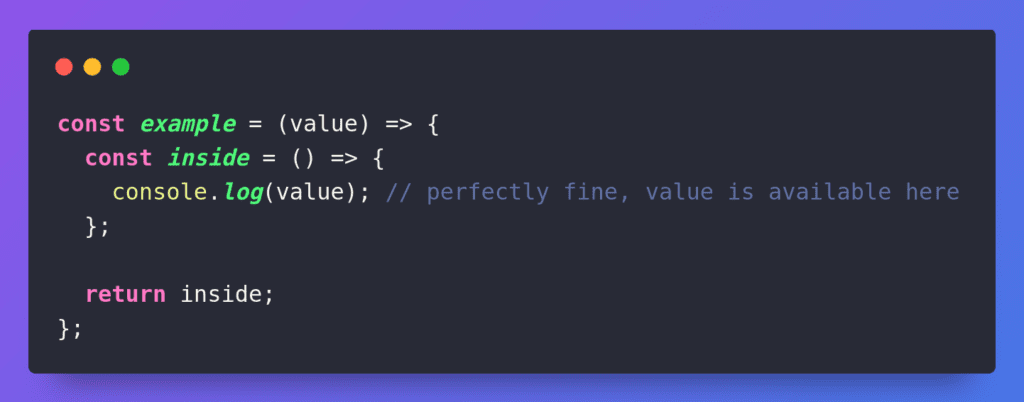
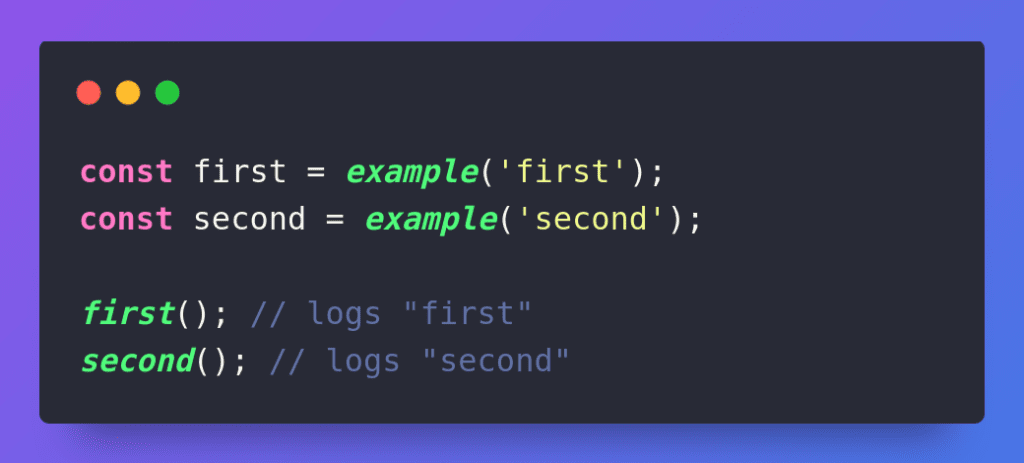
We call our example
function with an argument and store the result in a variable. The variable is a reference to a function declared inside example
. Now this forms a closure. As long as this variable exists, the parameter 'first'
we passed will remain frozen and the closure created will have access to it.
The same story for the second
variable.
Stale Closure Problem
The Stale Closure problem in JavaScript refers to a situation where a closure retains a reference to a variable from an outer function even after that outer function has completed and its execution context should theoretically be gone. This can lead to unexpected behavior, as the closure still holds the “stale” (outdated or no longer valid) value of the variable.
In React, it occurs when a closure captures a variable from a previous render, creating a reference to an outdated state or prop value. This can result in components not behaving as expected and developers scratching their heads in confusion.
Unraveling the Mystery
Now with all this in our head, we shall solve our original problem.
The Problem in our example is as our HeavyComponentMemo
component only renders when there is a change in the title
prop, it will not receive the newly created closure. Instead, it will receive the same closure which passed initially when rendered.
As I mentioned earlier, all the data around the closure is frozen. So the closure created at the time of mounting will see the input
value at the time it mounted which is undefined
.
Now we need an onClick
callback that doesn’t re-render on every render and should able to access the latest state without re-creating itself.
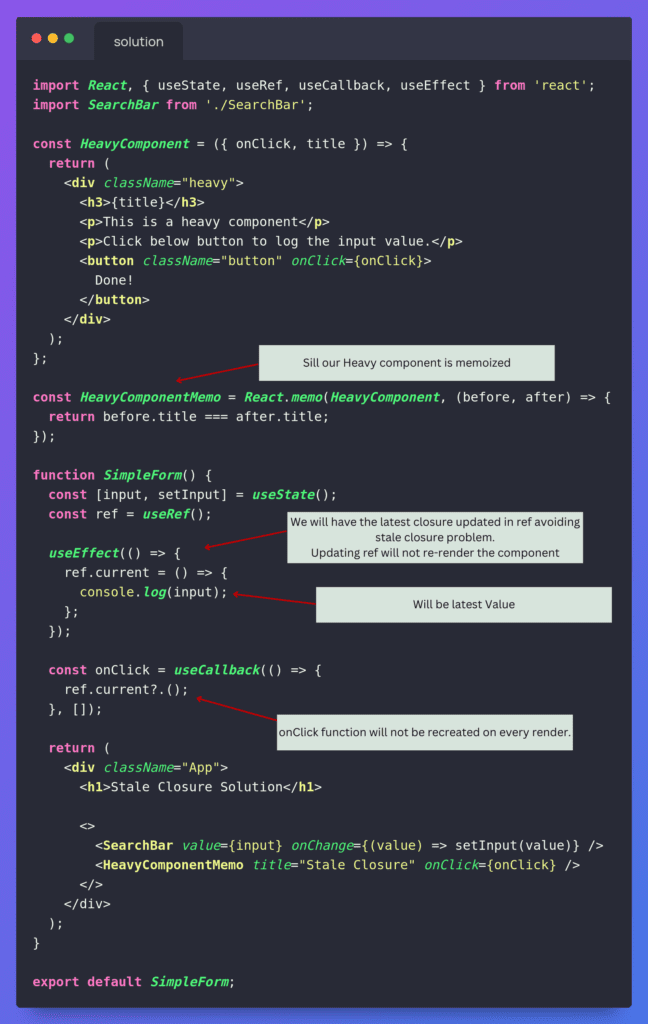
With this, we’ve achieved what we have intended, the user can type any text in the SearchBar
without any lag, HeavyComponent
is properly memoized, and doesn’t re-render on state change. The onClick
callback will always have the latest value. We can safely send everything we need to the server now.
If you want to explore the solution firsthand, check out the codesandbox link provided here.
How to Identify the Stale Closure Problem
Spotting the Stale Closure Problem can be tricky, but fear not! Here are some telltale signs to watch out for:
- Unexpected Behavior: If your component is displaying behavior that doesn’t align with your expectations, there’s a chance that stale closures are at play.
- Console Warnings: Keep an eye on your console for warnings related to stale closures. React is usually good at pointing out potential issues, so pay attention to any red flags it raises.
- Inconsistent Data: If your component is displaying inconsistent data or not updating as expected, it’s worth investigating whether stale closures are interfering with the state.
Solutions and Best Practices:
- Using
let
orconst
Inside Loops: Ensure you uselet
orconst
instead ofvar
when declaring variables inside loops. This helps avoid the Stale Closure Problem caused by variable hoisting. - Using Function Parameters: Pass variables as parameters to functions to create a local copy within the function scope.
Summary
Understanding and conquering the Stale Closure Problem in React is crucial for building robust and bug-free applications. By keeping an eye out for unexpected behavior, console warnings, and inconsistent data, you can identify and address stale closures effectively.
- Every time a function creates another function inside it, it forms a closure.
- Every function we create inside a React component is a closure because every React component is just another function, even the hooks we declare.
- When creating the closure, it freezes all the variables and constants around the enclosing scope/environment.
- If we need to get the updated data which is frozen, we need to re-create the closure.
- In React, we can make use of
Refs
which is persistent in nature to escape Stale Closure traps.
I hope all of this made sense. Let me know in the comments if you’ve faced this issue before and share how you’ve resolved it.
References
https://react.dev/reference/react/memo#specifying-a-custom-comparison-function
https://www.w3schools.com/js/js_function_closures.asp
https://react.dev/reference/react/useRef
https://adevnadia.medium.com/fantastic-closures-and-how-to-find-them-in-react-d81f000919d2
Thanks for reading!!
To learn more about Engineering topics visit – https://engineering.rently.com/
Senior Software Engineer,
Rently Software Development Private Limited,
Coimbatore.