So you might have guessed from the title we are going to talk about Infrastructure as code. So if you don’t know what Infrastructure as code(IaC) yet. it’s my pleasure to play cupid and introduce you to my friend here ( because I am pretty sure you are gonna love it )
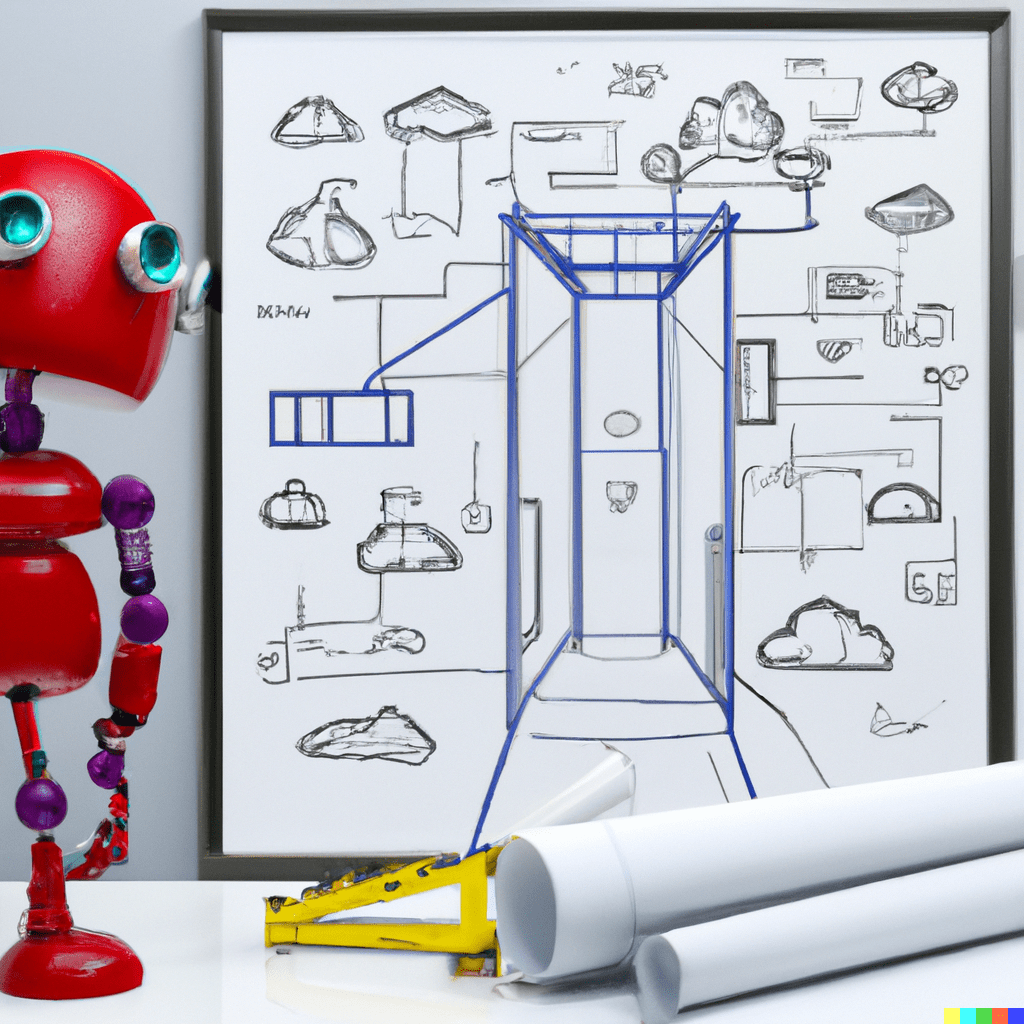
It’s better to start with an example and we will build it from there.
The Requirement
You have to build a file-processing pipeline. Where the file that gets uploaded to an S3 bucket gets processed using a lambda function. Fairly simple right? ( famous last words )
You log in to the AWS dashboard and click here, click there, and give resources some sensible name. Add proper policies, and upload code for lambda. Voila, you are done, phew !!! . everything works and you get a pat on your back.
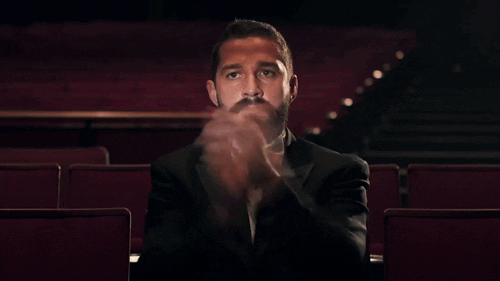
The Challenge
Now you have been asked to deploy this in another environment. You follow all the steps as you did before, done. You test the setup and for some reason, it’s not working. Then you realize that you forgot to add proper policies, instead of reading you gave the lambda write access to the bucket. So you go back and change, and now it works.
So This is a common issue that can ( and definitely will ) happen if you are creating AWS resources manually via the AWS web dashboard. Sometimes a simple miss-click can be fatal.
The Solution
So what if instead of going to the dashboard when you want to create cloud resources, You have a set of tools which allows you to define resources in a structured manner While being able to version control and deploy it at will? That would be awesome right?
There are several tools allowing you to do it. I will introduce you to a few such offerings from AWS’s side.
This is a good article that will give you some good insight into the progression of IaC: https://www.infoq.com/presentations/history-infra-as-code/
AWS Cloud Formation: The OG of Infrastructure as Code
First up, we have AWS Cloud Formation, the OG of Infrastructure as Code. Cloud Formation has been around for a while now It’s pretty good. Cloud Formation is written in JSON or YAML and it uses a declarative syntax to describe the resources you want to create. It’s a solid tool and it gets the job done.
AWSTemplateFormatVersion: '2010-09-09' Resources: S3Bucket: Type: AWS::S3::Bucket Properties: BucketName: my-file-processing-bucket LambdaFunction: Type: AWS::Lambda::Function Properties: FunctionName: my-file-processor Runtime: nodejs12.x Code: S3Bucket: my-lambda-functions S3Key: file-processor.zip Handler: index.handler Role: !GetAtt IamRole.Arn Timeout: 30 Environment: Variables: BUCKET_NAME: !Ref S3Bucket IamRole: Type: AWS::IAM::Role Properties: AssumeRolePolicyDocument: Version: '2012-10-17' Statement: - Effect: Allow Principal: Service: - lambda.amazonaws.com Action: - 'sts:AssumeRole' Path: / Policies: - PolicyName: root PolicyDocument: Version: '2012-10-17' Statement: - Effect: Allow Action: - 's3:GetObject' Resource: !Sub 'arn:aws:s3:::${S3Bucket}/*'
If you are familiar with AWS, it’s going to be pretty easy for you to understand what this does and what resources it creates.
But as the complexity of the infrastructure grows the YML file will be hard to maintain. It is a struggle to write the perfect Cloud Formation template and hard to debug the JSON cloud formation template That’s why we’re here today, to see if there’s a better way.
The prodigy – AWS SAM
The next iteration of cloud formation was AWS SAM aka Serverless application model. SAM was created to help developers build serverless applications. While leveraging the power that the cloud formation template brings, SAM adds some extra functionality to help with serverless deployments making it a more developer-friendly solution for application deployment and management.
So for this article’s sake let’s forget that this exists and move on ( or else I will have to overly complicate this). 😁
The New Kid on the Block – AWS CDK
So What comes into your mind when you think “Code“? It’s obviously not JSON or YML, pfff!!!!. Those are markup languages, Who even calls them code? ( almost everyone actually 😬, but that’s not the point here ).
So what if you can harness the power of cloud formation templates by using “actual code“? Languages you are familiar with and love playing around with.
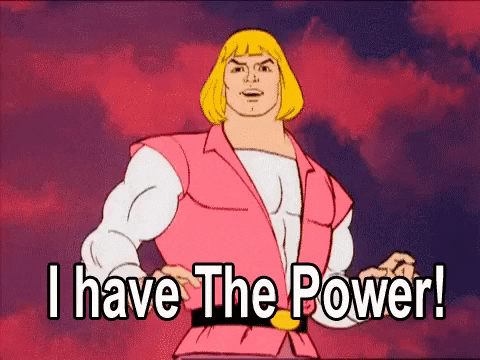
For that, there is AWS CDK ( Cloud development Kit ). CDK is different from Cloud Formation and SAM in a few key ways. First of all, CDK can be written in TypeScript, JavaScript, Python, Java, or C#. Which makes it much easier to write and debug. CDK uses imperative syntax, which means you can write code to describe your infrastructure, just like you would with any other programming language. This makes CDK much more powerful and flexible than Cloud Formation and SAM.
So if we want to create the same set of resources in AWS as we did with cloud formation, using CDK is much more imperative
import * as cdk from 'aws-cdk-lib';
import * as lambda from 'aws-cdk-lib/aws-lambda';
import * as s3 from 'aws-cdk-lib/aws-s3';
const app = new cdk.App();
const stack = new cdk.Stack(app, 'my-file-processing-stack');
const bucket = new s3.Bucket(stack, 'my-file-processing-bucket', {
bucketName: 'my-file-processing-bucket',
});
const myLambdaFunction = new lambda.Function(stack, 'my-file-processor', {
runtime: lambda.Runtime.NODEJS_12_
code: lambda.Code.fromBucket(bucket, 'file-processor.zip'),
handler: 'index.handler',
environment: {
BUCKET_NAME: bucket.bucketName,
},
});
bucket.grantRead(myLambdaFunction)
Boom, done !!.
For me, the CDK “code” looks much cleaner and readable. Even if you don’t know anything about AWS you can understand 70% of what we are trying to do here.
Other than being more approachable for beginners there are a lot of things CDK does that I feel are awesome.
Sensible defaults
When you define a resource, it has its set of default configuration objects set to sensible defaults based on common use cases and best practices, so we don’t have to worry about making mistakes.
So for example When creating a Lambda function using the AWS CDK, the CDK sets sensible defaults for things like the function’s memory and timeout. By default, a Lambda function created with the CDK will have a timeout of 30 seconds and 128 MB of memory ( It’s what we have in the AWS dashboard as well ).
Testcases
Yes, you might have guessed that right. CDK allows us to write test cases for the infrastructure we are deploying. Since CDK code is written using a programming language we can make use of the testing libraries that are available for that library to check if we are doing everything right even before we deploy the cloud formation template.
Crazy thing is that we can even deploy a testing stack that gets build first and tests the entire flow first and only make changes to production if all the test cases pass.
So for the above example stack, we can write a test case Which uploads a file to the s3 bucket and see if the lambda function gets called. ( mind blown! )
Automatic validation and Code Autocomplete
If we are using modern languages like typescript to build the CDK stacks. It allows us to leverage existing tools available for that language to make out life easier.
Automatic validation checks your code for syntax errors, logical issues, and configuration problems as you write it. If you are creating a resource that doesn’t exists. Made a typo, CDK got your back.
OOPS
Yep, that OOPS. You can create abstractions around AWS resources. So if you want a set of permission on a public bucket that gets created, Sure create a MyBucket the call which inherits from the base S3 Bucket class.
Read more on constructs here: https://docs.aws.amazon.com/cdk/v2/guide/constructs.html
Also, you can group your resources into Stacks and Combine them together. For example, If you need to pre-compile your TypeScript code to javascript before deploying to lambda? Sure, there is a built in construct that does it.
Regarding IaC, the AWS CDK offers several advantages over traditional tools such as CloudFormation templates or AWS SAM. So, why not take advantage of these benefits and make your life easier?
Thanks for reading!!
To learn more about Engineering topics visit – https://engineering.rently.com/
Get to know about Rently at https://use.rently.com/
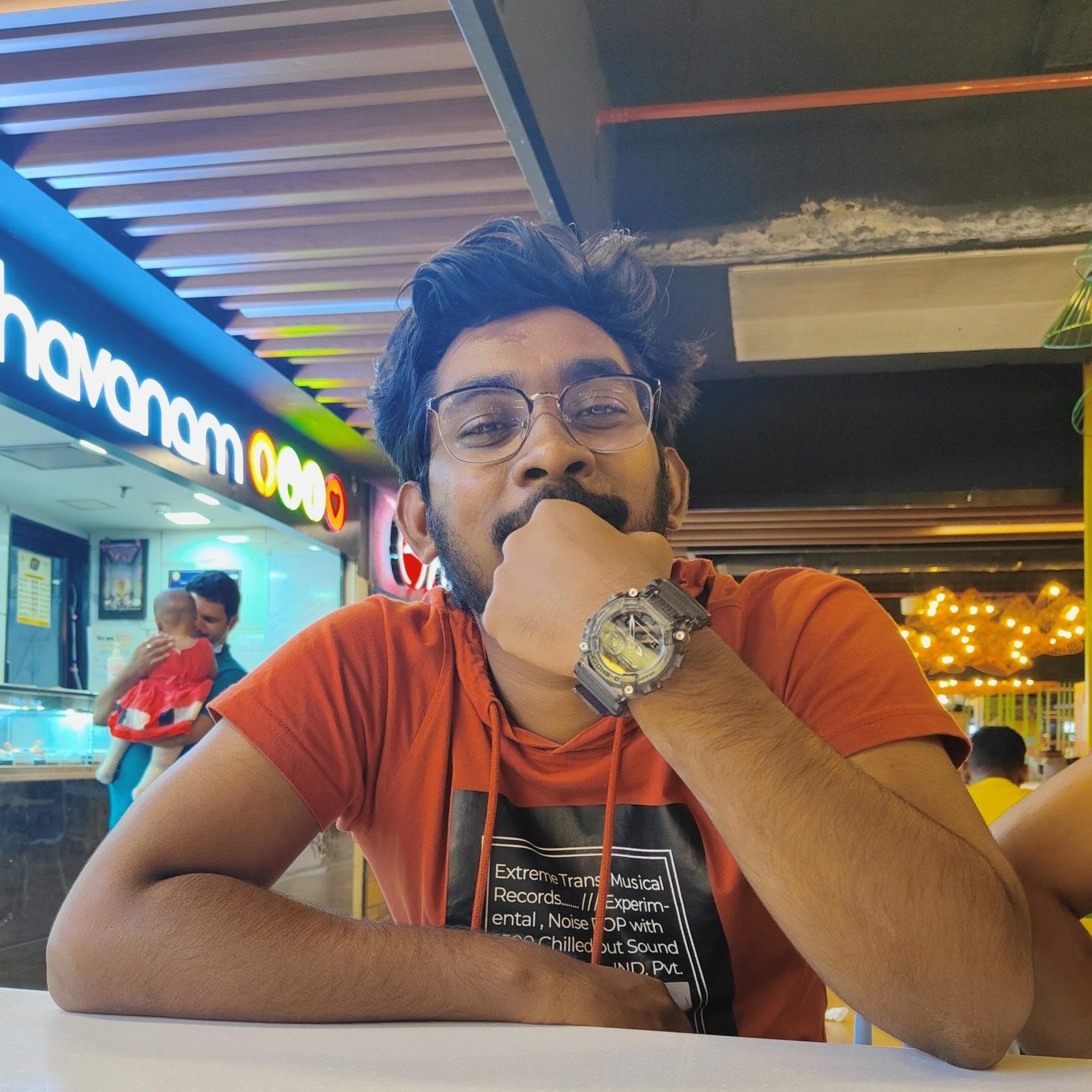
Senior software Engineer at Rently Architecture